When we launched in April, it was immediately clear that developers loved accessing their data directly from client-side JavaScript. Many people wondered, however, if data could ever be secured without running server-side code. “How do I secure my app?” was our most asked question.
We wanted to answer this right away, but security is so important that we didn’t want to announce anything until it was solid. Our requirements were clear: keep all of the parts that people love about Firebase — real-time updates, easy development, scaling — while adding enterprise-grade security. We’ve tested multiple approaches (with our awesome beta testers) and written a lot of code, and we’re finally ready to show you our Security API.
We’re excited to say that not only have we met our requirements, but we believe we’ve built the most flexible security model of any cloud data service.
The Big Picture
Building real-time apps is hard and scaling them is even harder. Firebase takes care of these complexities for you and lets you focus on building your app. Our new security model lets you build secure apps where clients talk directly to Firebase. This means that for many apps, you don’t need to write any server code, and it even makes running your own servers optional.
The Security API
The Firebase Security API consists of two key pieces:
1. Authentication
The authentication API lets you tell Firebase who a user is. We’ve designed this API to give you maximum flexibility, and we provide 3 easy methods for authenticating:
- Server-signed auth tokens (that you generate on your own servers)
- Our built-in Firebase Simple Login service. (This provides Email & Password, Facebook, and Twitter login out-of-the-box)
- Third-party services (like Singly)
Update (October 3, 2014): Firebase Simple Login has been deprecated and is now part of the core Firebase library. Use these links to find the updated documentation for the web, iOS, and Android clients.
2. Security & Rules
Security and Firebase Rules tell Firebase which operations are permitted for a specific user. You upload these rules to Firebase when you deploy your app, and we enforce them consistently whenever data is accessed.
The rules are where the new API shines.
The rules themselves are simple JavaScript-like expressions. This means that you don’t have to learn a new language to write them. They are also extremely flexible: you can compose your expressions using data already in a Firebase database, incoming data, auth credentials, current server time, and more.
Rules are stored as JSON on the Firebase servers. You can upload and edit them directly from our freshly redesigned graphical debugger, now called Forge:
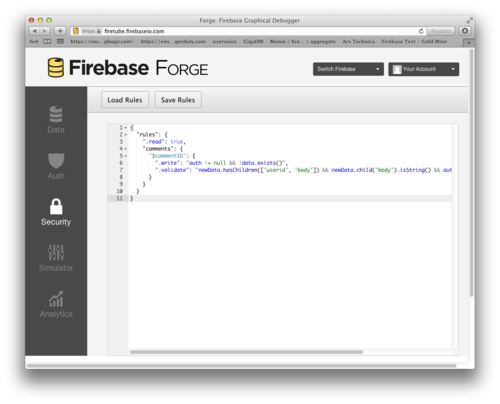
Rules come in three flavors: read and write rules allow or deny operations when reading or writing, and validate rules enforce a specific schema on your data.
Below is an example set of rules. These rules were written for a website with user accounts, where anyone can read data, but users may only edit their own profiles. The rules also ensure that user data conforms to a specific schema:
{
"rules": {
"users": {
"$user": {
".read": "true",
".write": "$user == auth.username",
".validate": "newData.hasChildren([name])",
"name": {
".validate": "newData.hasChildren([first, last])",
},
"age": {
".validate": "newData.isNumber() && newData.val() >= 0"
},
"about_me": {
".validate": "newData.isString()"
}
}
}
}
}
The Firebase rules language is designed for performance and scale; its expression-based rules provides fast, predictable execution times and allow Firebase to optimize and cache the results of those expressions.
An important benefit of the Firebase security model is that it places all of your security logic in one place rather than sprinkling it around your code. Firebase then ensures that your logic is enforced consistently across all parts of your app, regardless of how data is accessed. This makes it very easy to reason about your app’s security or do formal security audits. The rules also allow for static analysis, so mistakes can be caught immediately when new rules are uploaded.
For more rules details, see our documentation.
Implementation Details
When it comes to security, details matter, so we’ve taken great care with the little things. Specifically, Firebase:
- Supports SSL on all clients
- Uses 2048 bit keys for our SSL certificates
- Signs authentication tokens with SHA256 HMAC signatures
- Uses BCrypt for password storage
- Uses the JSON Web Token Standard for credentials
(The list is actually much longer, but hopefully you get the idea). Our goal is to take care of all of the complex but mundane details of securing your app so that you can focus on your application logic and your customers (rather than, say, reading about the latest hash function vulnerabilities).
More Goodies
Security is not the only thing we’re announcing today! We’re also launching:
- firebase.github.io - We’ll put code snippets, full examples, and libraries here that you’re free to fork, modify, and use in your apps. We’ll continue adding more content over time, so keep checking back.
- Security Simulator - Our security simulator lets you test our Security and Firebase Rules while building your app. It lets you simulate various authentication methods and data operations, and it provides helpful feedback to help you track down potential problems. You can find the simulator by going to the URL of your Firebase database.
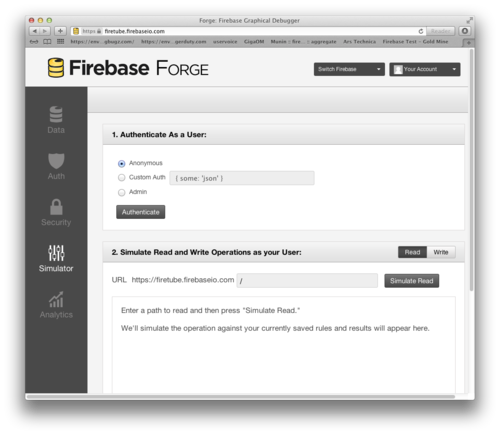
Wrapping Up
We’re really excited to show you our security features. The whole team has put an incredible amount of work into making them easy-to-understand and easy-to- use, qualities we strive for in everything we release. We hope you will use these new features and give feedback to help us improve. If you don’t have a beta code yet, you can request one here. Also look out for our public beta launch soon.
Here are some resources you can use to get started
- Read the Security Quickstart Guide, Authentication and Security and Firebase Rules docs
- See security examples on firebase.github.io
- Login to see the new graphical debugger, Forge.
- If you’re new to Firebase, try our 5 minute tutorial.
- Follow us on Twitter
Now go and build something awesome!
Enjoy the holidays and happy coding!