The best way to build a hybrid app is to deal with the underlying details of Cordova as little as possible. For this, Ionic is your best friend. Ionic abstracts the difficult parts of hybrid development into an easy to use SDK.
But, there still is one area of difficulty. Social login. Logging in with a social provider requires a popup or a redirect. The problem is, this doesn’t exist in the native world. It’s okay though, there’s another way, and it’s easy.
Setup
For this tutorial, 80% of the battle is just setting up. The actual code writing part is much easier.
If this is your first rodeo with Ionic, here’s a few steps to get you up and running. If you’re a seasoned Ionic veteran, you can skip this section.
Make sure your machine’s version of Node.js is above 4.x
. Using npm, download the following dependencies:
npm i -g ionic && cordova
npm i -g ios-deploy
After the install is done, you’ll create a new project.
Creating a project
Using the Ionic CLI, create a new project.
ionic start firebase-social-login tabs
One the setup is done, add both iOS and Android platforms:
ionic platform add android
ionic platform add ios
Installing dependencies
The last part of the setup is to add AngularFire and the InAppBrowser
plugin. They’ll be more on the InAppB`rowser plugin later.
ionic plugin add cordova-plugin-inappbrowser
ionic add angularfire
Now that everything is installed, let’s make sure the app is able to run.
Build and run
To build for either iOS or Android run the following command:
ionic build android
# or for ios
ionic build ios
Then run the emulator/simulator:
ionic emulate android
# or for ios
ionic emulate ios
You should see the default project running on the emulated device.
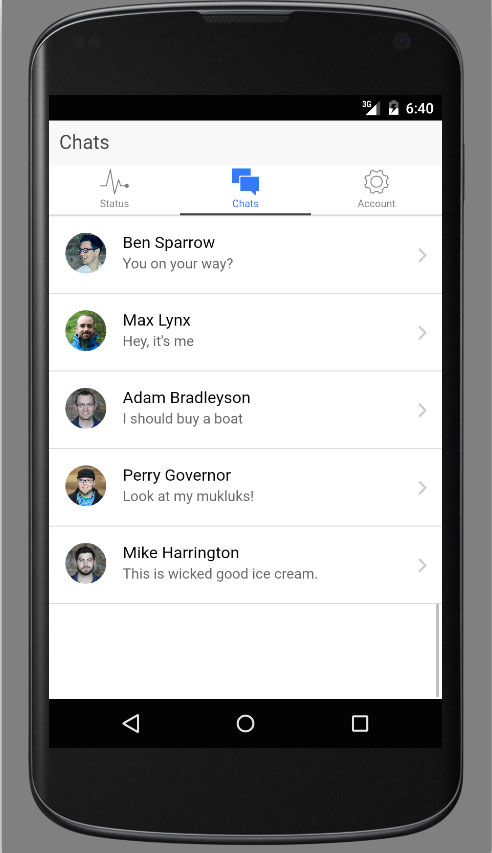
If you want to run it in the browser, then you should totally use Ionic labs.
ionic serve --lab
Ionic labs is a nifty tool that iframes both the iOS and Android styles side-by-side. Which makes for awesome Firebase tutorials, by-the-way.
With the build setup done, let’s get Firebase up and running.
Firebase Setup
Open up www/index.html
. Add the following scripts between Ionic and Cordova.
<!-- Firebase & AngularFire -->
<script src="lib/firebase/firebase.js"></script>
<script src="lib/angularfire/dist/angularfire.min.js"></script>
After the scripts have been added, open www/js/app.js
.
Declare AngularFire in the dependency array:
angular.module('starter', ['ionic', 'starter.controllers', 'starter.services', 'firebase'])
The Firebase setup is all taken care of. We’re ready to move on to the fun part, dependency injection!
Dependency Injection and Firebase
Add the following two lines of code right below the module declaration:
angular.module('starter', ['ionic', 'starter.controllers', 'starter.services', 'firebase'])
.constant('FirebaseUrl', 'https://ionicle.firebaseio.com/')
.service('rootRef', ['FirebaseUrl', Firebase])
The rootRef
service is a neat trick to inject a reference into any Angular service, factory, controller, provider, or whatever. You’ll use this in the next step, when setting up authentication.
Authentication setup
Open up www/js/services.js
, and add the following code:
function Auth(rootRef, $firebaseAuth) {
return $firebaseAuth(rootRef);
}
Auth.$inject = ['rootRef', '$firebaseAuth'];
Make sure to declare this function as a factory in the services
angular module:
.factory('Auth', Auth);
This style of code is based off the Angular Styleguide, check out the Github repo for more details.
In the code above, you’re simply injecting the rootRef
and $firebaseAuth
services. Since rootRef is a Firebase database reference, it is passed into the $firebaseAuth
service, and returned from the function. The $inject
property is a shorthand for declaring dependencies to work with minification.
That’s all the authentication setup needed. It’s time to create an API key with a social provider.
Obtaining a Social API Key
Firebase authentication allows you to login users to your apps with social providers like Google, Twitter, Facebook, and Github.
This tutorial uses Google, but you can use another if you’d like.
Social login with Firebase requires you to get a set of API keys from a provider. See the Firebase documentation on User Authentication for more details on getting a key and setting it up in the dashboard.
Once you’ve added an API and a Secret key to the Firebase App Dashboard, let’s create the login page.
Controller
To create the login page, you need three things: a controller, a view, and a route.
To create the controller, open www/js/controllers.js
and add the following snippet:
function LoginCtrl(Auth, $state) {
this.loginWithGoogle = function loginWithGoogle() {
Auth.$authWithOAuthPopup('google')
.then(function(authData) {
$state.go('tab.dash');
});
};
}
LoginCtrl.$inject = ['Auth', '$state'];
Then register the controller with the module:
.controller('LoginCtrl', LoginCtrl);
The LoginCtrl will be used with controllerAs
syntax. This means you attach methods to the controller using this
rather than $scope
. The controller has a single method, loginWithGoogle
, that will move the user to the 'tab.dash'
route once they’re authenticated.
View
The view couldn’t be simpler. Underneath www/templates
, create a login.html
file and add the following code:
<ion-view view-title="Login">
<ion-content>
<div class="padding">
<button class="button button-block button-assertive" ng-click="ctrl.loginWithGoogle()">
Google
</button>
</div>
</ion-content>
</ion-view>
The view uses a few components from Ionic’s SDK, like the “assertive” button. When the button is tapped, the loginWithGoogle()
method gets called.
That’s it for the view, let’s move onto the router.
Router
Open www/js/app.js
, and find the .config()
function. The .config()
function injects the $stateProvider
, which is used to tell the app which controllers to use for which routes.
Add the following route below:
.state('login', {
url: '/login',
templateUrl: 'templates/login.html',
controller: 'LoginCtrl as ctrl'
})
Notice that the route sets up the controller property to use controllerAs
syntax. This is what allows you to use the ctrl
variable in the login.html
template.
Using the InAppBrowser plugin
To use the InAppBrowser
plugin, do absolutely nothing. Yep, by simply installing the plugin, everything is handled for you.
The problem is, that on mobile there is no analogous “popup” view. The authWithOAuthPopup()
method uses window.open()
to to open up a new popup window. When this happens, Cordova won’t know what to do. The InAppBrowser
plugin fixes this by showing a web browser in your app when window.open()
is called.
So you can move on, because there’s nothing left to do here.
Build and run, again
Build and run the app for the emulator/simulator. You should the a basic login view. Tap the button to login. A browser window should popup, and let you login with a social account. After the authentication process completes, the app should move onto the dashboard view.
Get the code, and give it a star
Check out the completed app on Github. And, if you’re feeling generous, we would love a star. Feel free to fork the repo, and even send in a PR if you want.
Still having trouble?
If you’re running into issues, open up a question on Stackoverflow, we closely monitor the Firebase tag, or drop a post in our Google Group.