In this series of posts we cover the features of Firebase. This time we’ll talk about the most classic of Firebase features: the Realtime Database.### History The Realtime Database was the first feature we launched for Firebase, over four years ago. Cloud-hosted JSON databases were still somewhat new at the time, so it immediately stood out. What made the Firebase database truly unique was its ability to synchronize data between the different clients instantly, with a single, simple to use API.
The Realtime database hides the complexity of building applications behind one consistent programming interface. This made it easy to build realtime, collaborative applications.### What is it? The Realtime Database is really just one big JSON object that you manage in realtime. This means it’s really just a tree of values.
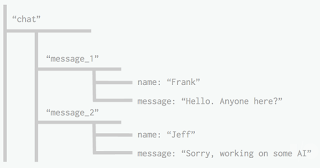
This allows for simple, flexible data modeling. The database is schemaless, which means you don’t have to commit to a fixed structure early on. But, if you’re worried about data validation, the Realtime Database comes with a server enforced rules language that allows you to validate the data structure of each write to the database.
The truly unique aspect of the Firebase database is in its realtime synchronization. Most traditional databases make you work with a request/response model. You write a query, then ask the database to give you the results for that query. If you need the results again, or want to check for updates to the results, you execute the query again.
Our database is different. Our database tells you when changes occur. You say to our database: if something changes at this location in the tree, notify me. This makes it simple to monitor changes and to keep these changes in sync across all users.
Realtime Events
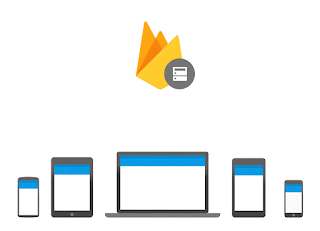
With a single API, the Firebase database provides your app with both the current value of the data and any updates to that data.
iOS
refHandle = postRef.observeEventType(FIRDataEventType.Value, withBlock: { (snapshot) in
let postDict = snapshot.value as! [String : AnyObject]
// …
})
Web
firebase.database().ref('posts/' + postId).on('value', function(snapshot) {
var post = snapshot.val();
// …
});
Android
mPostReference.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
// Get Post object and use the values to update the UI
Post post = dataSnapshot.getValue(Post.class);
// …
}
@Override
public void onCancelled(DatabaseError databaseError) {
// Getting Post failed, log a message
Log.w(TAG, "loadPost:onCancelled", databaseError.toException());
// …
}
});
Your app then displays the data in its user interface and allows the user to manipulate it. It’s that easy.### What happens when you’re offline.
Ever used an app that became unresponsive the very moment you lost network connection? Most developers want their app to work despite the network condition. That’s why the Firebase database keeps an internal cache of all data that is being shown in the app. When the network connection temporarily drops, the app keeps working from the cache. And while the connection is lost, the Firebase data client keeps a queue of local write operations. Your app continues to work and remains responsive, all without having to write any extra code.
The power of the Firebase platform is in the integration of all our features. We’re adding more integrations, but these are a few example of the integrations that you can already use. Allow your users to sign in to your app, then secure access to their data based on their identity. Store the user’s files in Firebase Storage, then use the realtime database to synchronize the status and availability to all other users of the app. Track what files are most popular with Firebase Analytics and invite other users to collaborate on those files. The possibilities are almost endless and it’s never been easier to build an app than with Firebase.