Firebase Auth is a secure authentication system that allows users to sign up and sign in for your application. It allows you to use federated identity through providers, such as Facebook, Twitter, and of course - Google. When doing this, your users will demand a rich user experience, and the burden of implementing this will fall on you as a developer. In addition, creating apps that allow for sign in involves a lot more than just signing in — there are many other user flows, such as choosing from multiple accounts, signing up for new accounts, resetting passwords and more. This can be a lot of work!
Luckily, the open-source FirebaseUI libraries make all of this really easy. In this post, you’ll take a look at building a simple web site that allows for sign in and sign up. You’ll use two providers: The built-in Email/Password on Firebase, and federated identity using Google Sign-In.
Getting Started
Creating a Firebase App
To use FirebaseUI, you need a Firebase app or site, and you created these on the Firebase Console. From here, select ‘Authentication’ on the left (1).
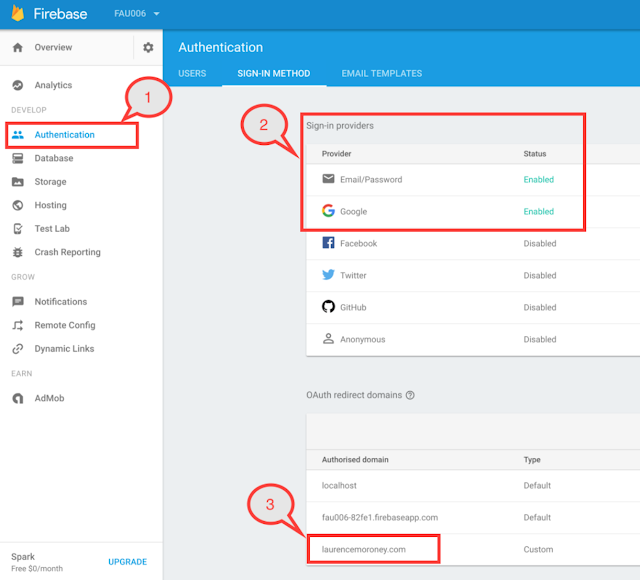
When you select ‘Sign-In Method’ at the top, you’ll be given a list of Sign-in providers. Choose ‘Email/Password’ and ‘Google’ (2). Make sure they’re enabled.
Additionally, if you are going to host these pages on your own domain, make sure the domain is added to the list of OAuth redirect domains at the bottom of the screen. (3).
Now, back on the Overview screen for your project, click ‘Add Firebase to your Web app’, and you’ll see a popup like this:
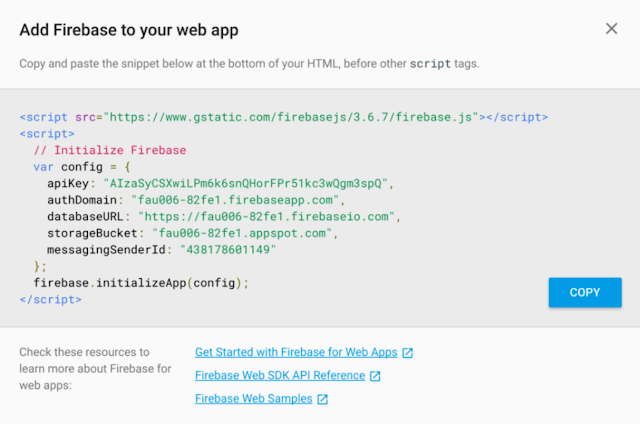
This contains all the code you need to initialize Firebase on your web site. Let’s take a look at a simple 2-page web site next: one page for signing in, and one page for after you’ve signed in.
A simple site with Sign In
I’ve built and hosted a simple site using FirebaseUI Auth here. The first page you see when you go to this site looks like this:
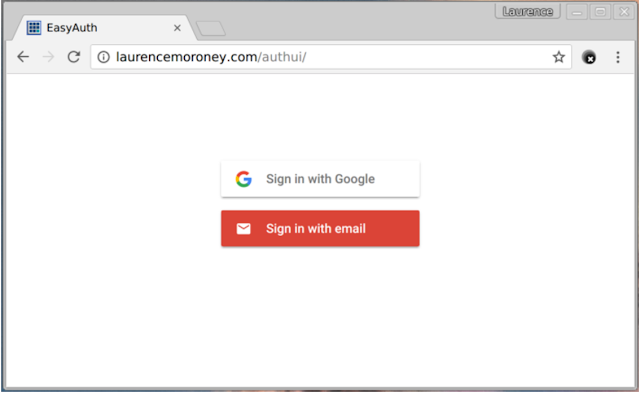
As you can see, it’s pretty straightforward, with two sign-in options — Google and Email, matching what we configured in the Firebase console.
Clicking Sign-In with Google can have multiple effects based on the context:
- If you’ve signed in previously on this browser, you’ll be taken straight back into the site
- If you have multiple Google accounts, you’ll be given the account chooser, with the choice to pick a different account, or create a new one, like this:
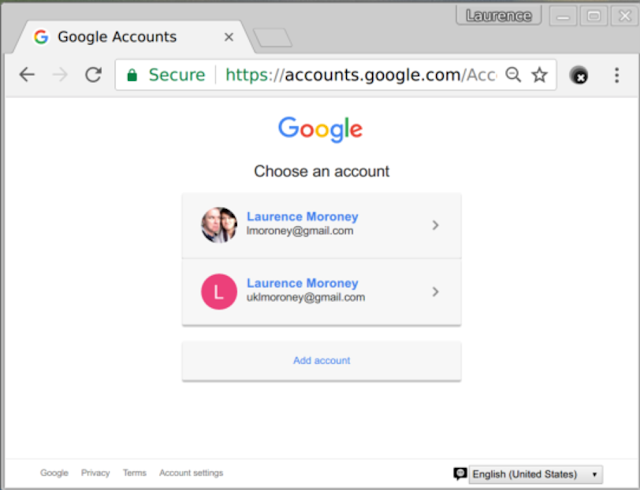
If you haven’t signed in on this browser, and don’t have a Google account, you’ll be given the option to enter one, or create a new one, like this:
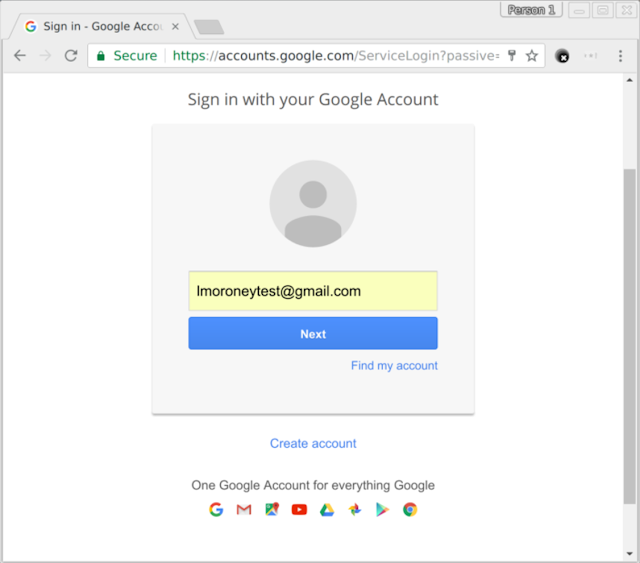
Clicking on the Create account link will take you through the standard user flow for creating an account, which will return you to your site to sign in when you’re done.
Similarly when using Email/Password auth, you’ll get the full user flow.
- If you’ve signed in with an Email/Password combination before, and you’re the only user, you’ll go straight into the site.
- If you’ve signed in with an Email/Password combination before, but aren’t the only user, you’ll get a list of accounts to choose from, like this:
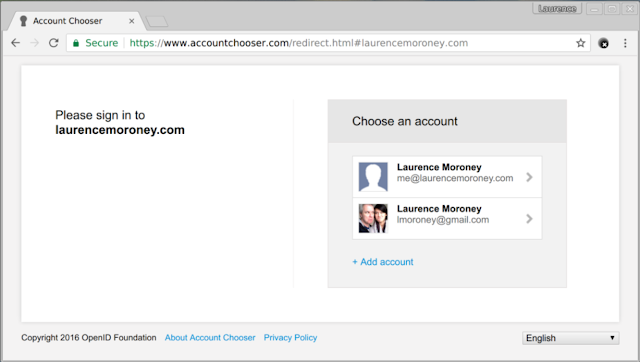
This also gives you the facility to add a new account, and doing so creates a new account on firebase that you can use to sign in in future. There are many scenarios, with a very complex flow. To see a detailed flowchart of these, click here.
Implementing
So let’s look at the code needed to implement this!
Coding the Log In Page
Here’s the full source code for the Log In page you’ve seen in this post. You’ll see that there are two highlighted blocks. The first is the initialization code that you got from the Firebase console earlier. The second uses the FirebaseUI open source code to create the user interface widgets.
The things to note in the second block are the signInSuccessUrl
parameter, which is the address of the page to redirect to once the sign in is successful. Also, note the signInOptions
setting where Google Sign-In and Email Sign-In are configured.
<!DOCTYPE html>
< html lang="en">
< head>
< title>EasyAuth</title>
<meta charset="UTF-8">
</head>
<!-- Below is the initialization snippet for my Firebase project. It will vary for each project -->
<script src="https://www.gstatic.com/firebasejs/3.6.4/firebase.js"></script>
<script>
// Initialize Firebase
var config = {
apiKey: "AIzaSyAPtNmUso5tA8d83vaJlgDHA_4C7HEgYNY",
authDomain: "authui-6818f.firebaseapp.com",
databaseURL: "https://authui-6818f.firebaseio.com",
storageBucket: "authui-6818f.appspot.com",
messagingSenderId: "596916061379"
};
firebase.initializeApp(config);
</script>
<!-- The code below initializes the sign-in widget from FirebaseUI web. -->
<script src="https://cdn.firebase.com/libs/firebaseui/1.0.0/firebaseui.js"></script>
<link type="text/css" rel="stylesheet" href="https://cdn.firebase.com/libs/firebaseui/1.0.0/firebaseui.css" />
<script type="text/javascript">
var uiConfig = {
signInSuccessUrl: 'loggedIn.html',
signInOptions: [
// Specify providers you want to offer your users.
firebase.auth.GoogleAuthProvider.PROVIDER_ID,
firebase.auth.EmailAuthProvider.PROVIDER_ID
],
// Terms of service url can be specified and will show up in the widget.
tosUrl: '<your-tos-url>'
};
// Initialize the FirebaseUI Widget using Firebase.
var ui = new firebaseui.auth.AuthUI(firebase.auth());
// The start method will wait until the DOM is loaded.
ui.start('#firebaseui-auth-container', uiConfig);
</script>
<!-- Include a simple background image & and title -->
<div></div>
<body>
<h1 align="center" style="color:white;">Firebase Auth Quickstart Demo</h1>
<div id="firebaseui-auth-container"></div>
</body>
</html>
Note that this is all the code you need to implement to get the user flows mentioned earlier. Everything is encapsulated in the open source library.
Handling the sign in event
The Signed-In page will need the first block of code in order to ensure that it uses Firebase, and then, when the onAuthStateChanged
event fires, you know the user is signed in, and you can query their exposed metadata.
Here’s the code:
<!DOCTYPE html>
<html lang="en">
<head>
<title>EasyAuth</title>
<meta charset="UTF-8">
</head>
<!-- Below is the initialization snippet for my Firebase project. It will vary for each project -->
<script src="https://www.gstatic.com/firebasejs/3.6.4/firebase.js"></script>
<script>
// Initialize Firebase
var config = {
apiKey: "AIzaSyAPtNmUso5tA8d83vaJlgDHA_4C7HEgYNY",
authDomain: "authui-6818f.firebaseapp.com",
databaseURL: "https://authui-6818f.firebaseio.com",
storageBucket: "authui-6818f.appspot.com",
messagingSenderId: "596916061379"
};
firebase.initializeApp(config);
</script>
<body>
<!-- A simple example script to add text to the page that displays the user's Display Name and Email -->
<script>
// Track the UID of the current user.
var currentUid = null;
firebase.auth().onAuthStateChanged(function(user) {
// onAuthStateChanged listener triggers every time the user ID token changes.
// This could happen when a new user signs in or signs out.
// It could also happen when the current user ID token expires and is refreshed.
if (user && user.uid != currentUid) {
// Update the UI when a new user signs in.
// Otherwise ignore if this is a token refresh.
// Update the current user UID.
currentUid = user.uid;
document.body.innerHTML = '<h1> Congrats ' + user.displayName + ', you are done! </h1> <h2> Now get back to what you love building. </h2> <h2> Need to verify your email address or reset your password? Firebase can handle all of that for you using the email you provided: ' + user.email + '. <h/2>';
} else {
// Sign out operation. Reset the current user UID.
currentUid = null;
console.log("no user signed in");
}
});
</script>
<h1>Congrats you're done! Now get back to what you love building.</h1>
</html>
That’s it!
And that’s it! Hopefully this primer will help you understand the power that you get with the FirebaseUI Auth libraries, and how they make it much easier for you to build web apps that sign in!