Update!
Cloud Functions for Firebase now supports native scheduling!
const functions = require('firebase-functions');
const admin = require('firebase-admin');
admin.initializeApp();
exports.scheduledFunction = functions.pubsub.schedule('every 5 minutes').onRun((context) => {
console.log('This will be run every 5 minutes!');
return null;
});
The article below predates this feature and is useful if you want to use AppEngine. However, we recommend using the scheduled functions feature provided by Cloud Functions.
Cloud Functions are a great solution for running backend code for your Firebase app. You can write a function which is triggered by many different actions like user sign-ups, writes to the Realtime Database, changes to a Cloud Storage bucket, or conversion events in Firebase Analytics. Cloud Functions can also be triggered by some external sources, for example you could tie a Cloud Function to an HTTPS endpoint or a Cloud Pub/Sub topic.
Reacting to these events is very powerful, but you may not always want to react to an event - sometimes you may want to run a function based on a time interval. For example, you could clean up extra data in your Realtime Database every night, or run analysis on your Analytics data every hour. If you have a task like this, you’ll want to use App Engine Cron with Cloud Functions for Firebase to reliably trigger a function at a regular interval.
How to Schedule Functions with AppEngine
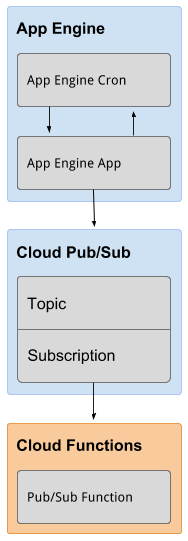
Cloud Functions for Firebase does not have any special support which allow us to utilize App Engine Cron to schedule events. In fact, the solution we’ll implement is nearly identical to the solution we recommend for doing reliable task scheduling on Google Compute Engine.
The trick is to create a tiny App Engine shim that provides hooks for App Engine Cron. These hooks will then push to Cloud Pub/Sub topics for each scheduled job.
We will then configure our Cloud Function to handle incoming messages on that Pub/Sub topic.
Although this solution is the preferred option for scheduling functions, it isn’t the only way you achieve this goal. If you’re interested in seeing an alternative method, you should check out the functions-samples repository which explains how to achieve a similar result using an external scheduling service.
Deploying the App Engine App
It just so happens that we’ve already written the App Engine app you’ll need to set up scheduled functions. It’s available in the firebase/functions-cron repo on Github.
By default this sample triggers hourly, daily, and weekly Cloud Pub/Sub ticks. If you want to customize this schedule for your app then you can modify the cron.yaml.
For details on configuring this, please see the cron.yaml Reference in the App Engine documentation.
Let’s get started!
1. Prerequisites
Install (or check that you have previously installed) the following tools.
2. Clone this repository
To clone the GitHub repository to your computer, run the following command:
git clone https://github.com/firebase/functions-cron
Change directories to the functions-cron directory. The exact path depends on where you placed the directory when you cloned the sample files from GitHub.
cd functions-cron
3. Deploy to App Engine
Configure the gcloud command-line tool to use your Firebase project.
gcloud config set project
Change directory to appengine/
cd appengine/
Install the Python dependencies
$ pip install -t lib -r requirements.txt
Create an App Engine App
gcloud app create
Deploy the application to App Engine.
gcloud app deploy app.yaml cron.yaml
Open Google Cloud Logging and in the right dropdown select “GAE Application”. If you don’t see this option, it may mean that App Engine is still in the process of deploying.
Look for a log entry calling /_ah/start
. If this entry isn’t an error, then you’re done deploying the App Engine app.
4. Deploy to Google Cloud Functions for Firebase
Ensure you’re back the root of the repository (cd ..
if you’re coming from Step 2)
Deploy the sample hourly_job
function to Google Cloud Functions
firebase deploy --only functions --project
Warning: This will remove any existing functions you have deployed. If you have existing functions, copy the example from functions/index.js into your project’s index.js
5. Verify your Cron Jobs
We can verify that our function is wired up correctly by opening the Task Queue tab in App Engine and clicking on Cron Jobs. Each of these jobs has a Run Now button next to it.
The sample functions we deployed only has one function: hourly_job
. To trigger this job, let’s hit the Run Now button for the /publish/hourly-tick
job.
Then, go to your terminal and run…
firebase functions:log --project
You should see a successful console.log
from your hourly_job
.
You’re Done!
Your cron jobs will now “tick” along forever. As we mentioned above, you’re not limited to the hourly-tick
, daily-tick
and weekly-tick
that are included in the App Engine app.
You can add more scheduled functions by modifying the cron.yaml file and re-deploying the app.