Are you looking for something new to learn this year? Then let me suggest TypeScript for development with Cloud Functions!
Not long ago, the Cloud Functions for Firebase team released an update to the Firebase CLI that makes it easy for you to write your functions in TypeScript, rather than JavaScript. The Firebase team encourages you to consider switching to TypeScript, but I can imagine you might be reluctant to learn a new language, especially if you’re already comfortable with JavaScript. The great news is that TypeScript offers you a bunch of benefits that are easy to start using today.
The language itself offers a bunch of features that make your code easier to read and write, and less error-prone:
- Static type checking (optional)
- Classes and interfaces
- Generics
- Enums
There’s also async/await from ECMAScript 2017, which helps you write asynchronous code more easily. The primary challenge with asynchronous code is management of promises, which is crucial to get right when writing Cloud Functions, but difficult to master. TypeScript makes that much easier.
But what I really want to dive into here is an excellent tool called TSLint that can check your TypeScript code for potential problems before you deploy to Cloud Functions. The Firebase CLI will prompt you to configure TSLint when you initialize Functions in a project using TypeScript, and we strongly recommend that you opt into it.
Enabling TypeScript and TSLint
When you opt into TypeScript and TSLint in your Firebase project structure, the Firebase CLI will add and modify some project files when you run firebase init
. First let’s take a look at functions/package.json
. In there, you’ll see the following key:
"devDependencies": {
"tslint": "^5.8.0",
"typescript": "^2.6.2"
},
This is where node pulls in TypeScript and TSLint for development. Notice that there are “devDependencies” that are separate from the normal “dependencies” that you use in your function code. devDependencies are only stored on your machine, and are made available as tools for development. They are not deployed with your code. Also in that file, notice there are two script definitions:
"scripts": {
"lint": "./node_modules/.bin/tslint -p tslint.json",
"build": "./node_modules/.bin/tsc"
}
These give you the ability to run npm run lint
and npm run build
on the command line from your functions directory. The first check your code with TSLint, and the second will build it with the TypeScript compiler.
The next file to look at is firebase.json
. This now has a predeploy hook that runs TSLint against your code, so if it has an error, the deploy will fail:
{
"functions": {
"predeploy": [
"npm --prefix $RESOURCE_DIR run lint",
"npm --prefix $RESOURCE_DIR run build"
]
}
}
The next file is functions/tsconfig.json
. This contains the configuration for the TypeScript compiler.
{
"compilerOptions": {
"lib": ["es6"],
"module": "commonjs",
"noImplicitReturns": true,
"outDir": "lib",
"sourceMap": true,
"target": "es6"
},
"compileOnSave": true,
"include": [
"src"
]
}
I won’t cover all the settings here, but it’s important to note that the compiler will look for source TypeScript files under functions/src
, and compile them to JavaScript into functions/lib
, with ECMAScript 6 compatibility, as required by Cloud Functions. Cloud Functions currently runs Node 6, which means it natively understand ES6 code.
Lastly, take a brief look at functions/tslint.json
. This lists all the rules that are observed when TSLint checks your code. You can add new rules here, or remove the rules you don’t want. I suggest leaving everything there, as the list of rules was curated by the Firebase team to help with writing functions. The “strict errors” whose values are set to true
will cause compile time errors if violated. The warnings below that will just complain about possible issues, and it’s the team’s opinion that you should look into resolving them.
Show me an example!
Alrightey. Take a look at this function that populates a property called createdAt
when a user node is created in Realtime Database. Do you see what’s wrong here?
export const onUserCreate =
functions.database.ref('/users/{uid}').onCreate(event => {
event.data.ref.update({ createdAt: Date.now() })
})
TSLint sees the issue, and its one of the most common mistakes made when writing functions. If you run npm run build
on this code, you’ll see this error in its output:
Promises must be handled appropriately
This error is triggered by the rule no-floating-promises. TSLint sees that event.data.ref.update
returns a promise, but nothing is being done with it. The correct way to deal with promises for database triggers is to return it:
export const onUserCreate =
functions.database.ref('/users/{uid}').onCreate(event => {
return event.data.ref.update({ createdAt: Date.now() })
})
If you’re using async/await, you can also declare the function async
and use await
to return it:
export const onUserCreate =
functions.database.ref('/users/{uid}').onCreate(async event => {
await event.data.ref.update({ createdAt: Date.now() })
})
Proper handling of promises is an absolute requirement when dealing with Cloud Functions, and TSLint will point out where you’re not doing so.
I want faster feedback!
I do a lot of Android development, and I’m accustomed to Android Studio linting my code as I type it. This is valuable because I get instant feedback about where things could go wrong. On the Firebase team, a bunch of us use VSCode for editing TypeScript, and it will use TSLint to give you instant feedback. The TSLint extension is easy to install.
Go to View -> Extensions. Type “TSLint” into the search box on the left. Find the TSLint extension, and click the Install button. After it’s installed, click the Reload button, and now your TypeScript will be marked with possible mistakes.
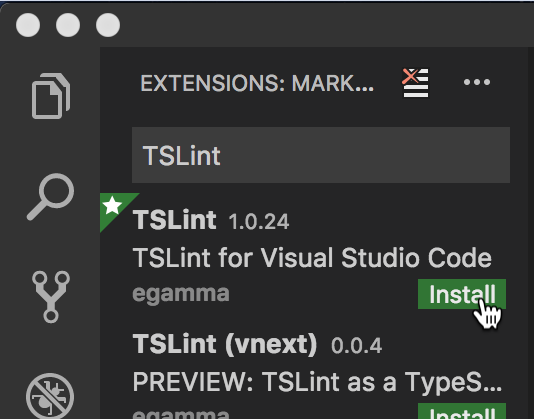
How about another example?
Here’s an HTTPS function that fetches a user document from Firestore using the Admin SDK. It looks OK and works OK:
export const getUser = functions.https.onRequest((req, res) => {
var uid = req.params.uid
const doc = admin.firestore().doc(`users/${uid}`)
doc.get().then(doc => {
res.send(doc.data())
}).catch(error => {
res.status(500).send(error)
})
})
But when viewed in VSCode with TSLint markers, you’ll see that it violates some best practices:
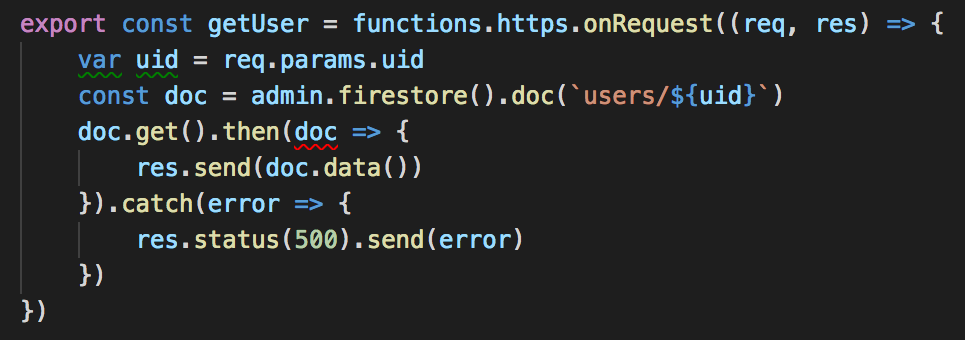
Notice the squiggly lines under var
, uid
, and doc
. If you hover the mouse over the marked code, you’ll see a message from TSLint:
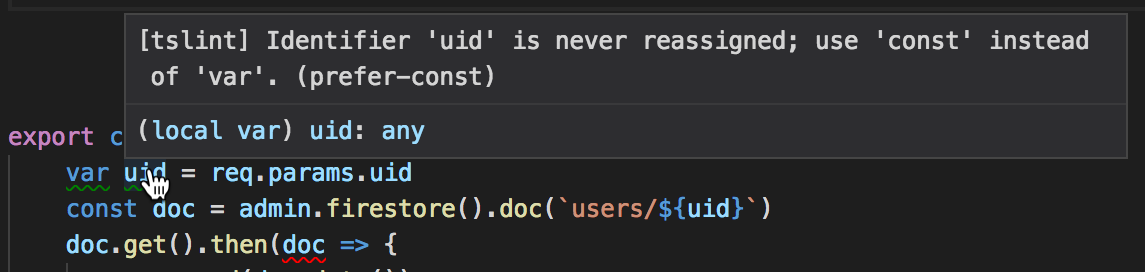
That’s TSLint using the prefer-const rule to tell you that it’s better to use const
instead of var
to declare values that don’t change. It’s good for the readability of your code, and also prevents someone from making an accidental change to it later on.
The squiggly line under doc
is TSLint using the no-shadowed-variable rule to point out that the doc
parameter in the function passed to then()
(a DeltaDocumentSnapshot object) is masking the doc
constant in the outer scope (a DocumentReference object), making the latter completely unavailable for use. While this is not really a bug here, it can lead to confusion about which doc instance is being referred to at any given moment. Renaming either instance resolves the issue.
Here’s a lint-free version of the same function:
export const getUser = functions.https.onRequest((req, res) => {
const uid = req.params.uid
const doc = admin.firestore().doc(`users/${uid}`)
doc.get().then(snapshot => {
res.send(snapshot.data())
}).catch(error => {
res.status(500).send(error)
})
})
This makes TSLint happy, which should make you happy, because you’re writing better code!
If you haven’t started learning TypeScript yet, 2018 is a fine time to begin. It’s easy to get started, because TypeScript is a strict superset of JavaScript, meaning that all your existing JavaScript code is already valid TypeScript. So you can simply rename your .js files to .ts and drop them into functions/src. Then, you can start using TypeScript language features as you wish. Let us know how it goes and shout out to @Firebase on Twitter.