In Firebase Crashlytics, you can view crashes and non-fatals by versions. Several of our customers take advantage of this filtering, especially to focus on their latest releases.
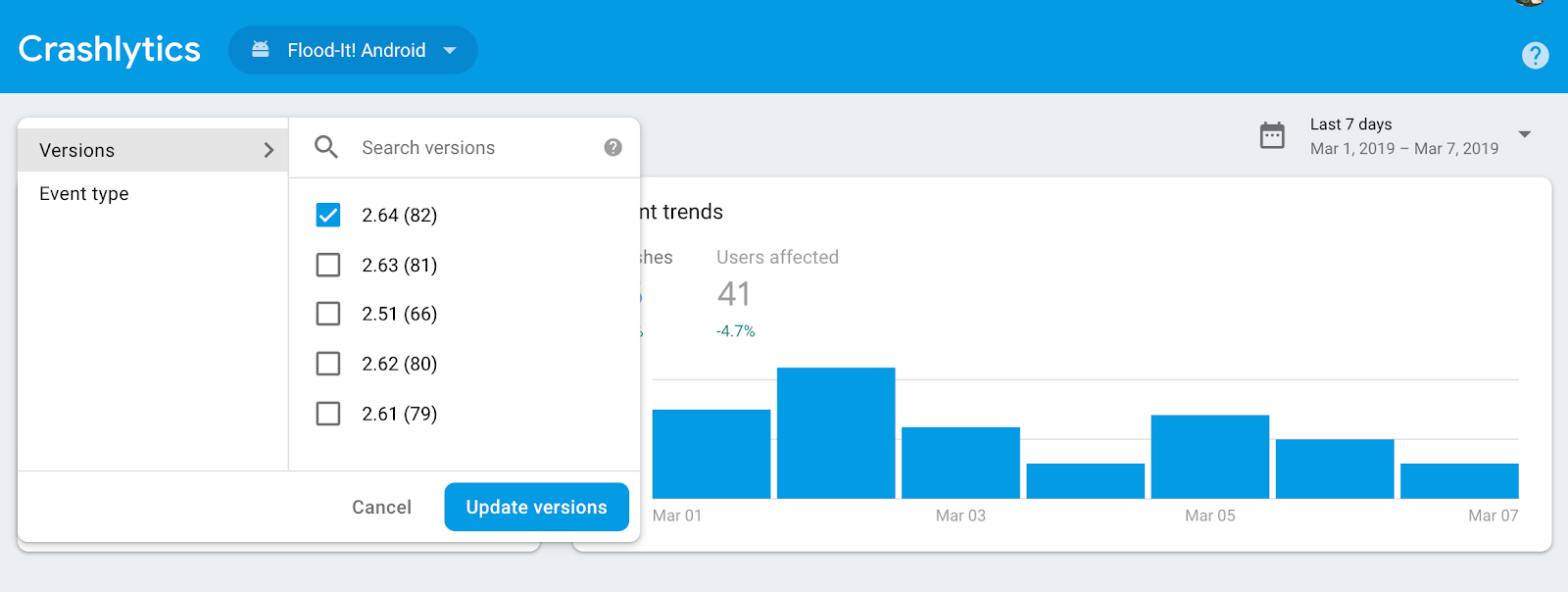
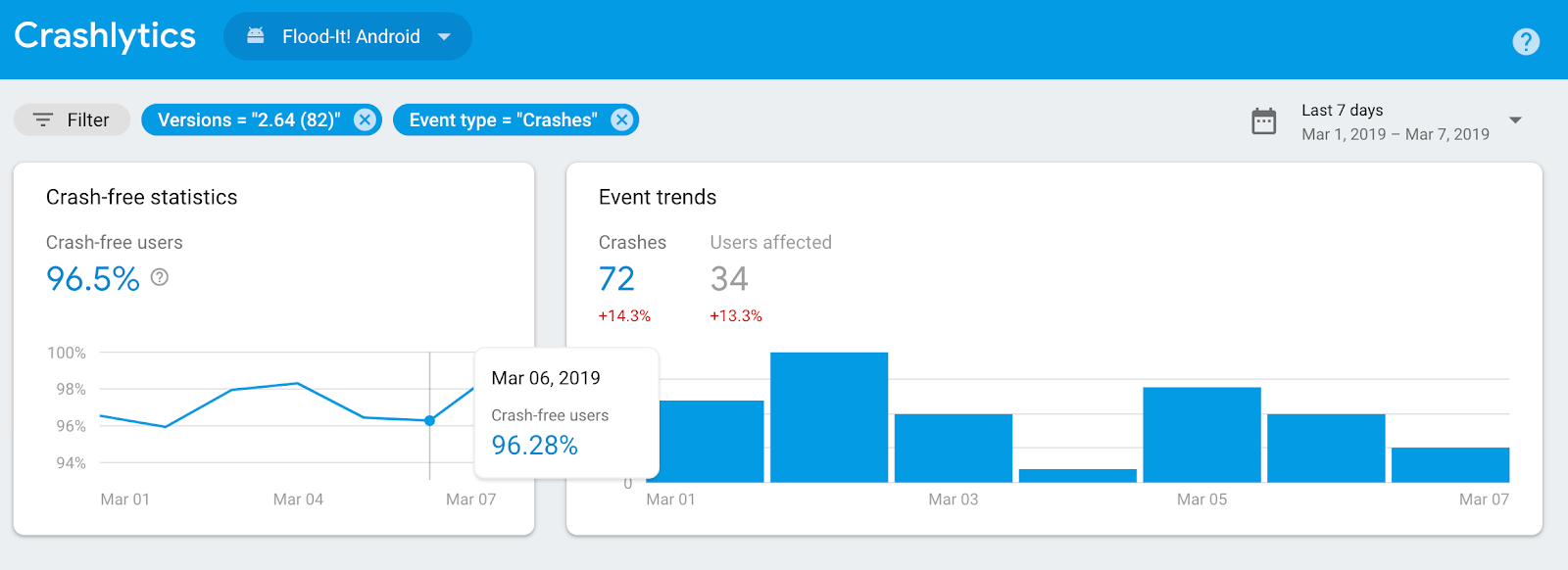
But sometimes too many versions can be a bad thing. Firebase Crashlytics - by default - shows you the last 100 versions we have seen. If you have a lot of debug versions created by developers, and by continuous Integration and deployment pipeline, you might soon start to not see the versions that really matter e.g., production builds.
So how do you make sure that this does not happen? Disabling Crash reporting initialization for debug builds is the simplest way of achieving this. Let’s explore how to do this on iOS and Android.
iOS
For iOS apps, first check if you are manually initializing Crashlytics (this happens for Fabric apps that were linked to a Firebase app).
If you use Swift, search for the line Fabric.with([Crashlytics.self])
in AppDelegate.swift. If this line is present, then you are manually initializing Crashlytics, otherwise you are using automatic initialization.
If you use ObjectiveC, search for the line [Fabric with:@[[Crashlytics class]]];
in AppDelegate.m. If this line is present, then you are manually initializing Crashlytics, otherwise you are using automatic initialization.
Apps with Manual initialization
For apps that are using manual initialization, you can just not initialize Crashlytics for DEBUG versions.
For Swift
#if !DEBUG
Fabric.with([Crashlytics.self])
#endif
For ObjectiveC
#if !DEBUG
[Fabric with:@[[Crashlytics class]]];
#endif
Apps that are auto initialized
Firebase Crashlytics apps are automatically initialized by Firebase. You can turn off automatic collection with a new key to your Info.plist
file:
- Key:
firebase_crashlytics_collection_enabled
- Value:
no
Then you can initialize it as shown in the examples above for Swift and ObjectiveC
Android
For Android apps, first check if you are manually initializing Crashlytics (this happens for Fabric apps that were linked to a Firebase app). Search for Fabric.with
in your project. If this line is present, then you are manually initializing Crashlytics. Otherwise, Crashlytics is being automatically initialized through Firebase.
Apps with Manual initialization
To disable Crashlytics in debug builds, you can make use of the BuildConfig.DEBUG
flag. Edit the Fabric.with statement you found previously, adding a check for the DEBUG flag:.
if (!BuildConfig.DEBUG) {
Fabric.with(this, new Crashlytics());
}
Apps that are auto initialized
Turn off Crashlytics initialization in your debug builds by creating a debug
folder in your src
directory and creating an AndroidManifest.xml with this snippet:
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
<application>
<meta-data
android:name="firebase_crashlytics_collection_enabled"
android:value="no" />
</application>
</manifest>
This snippet will be merged into the manifest of all of your debug variants, and will disable Crashlytics in those builds. If you’d prefer finer-grained control, you can use this approach for any variants you’d like to exclude.