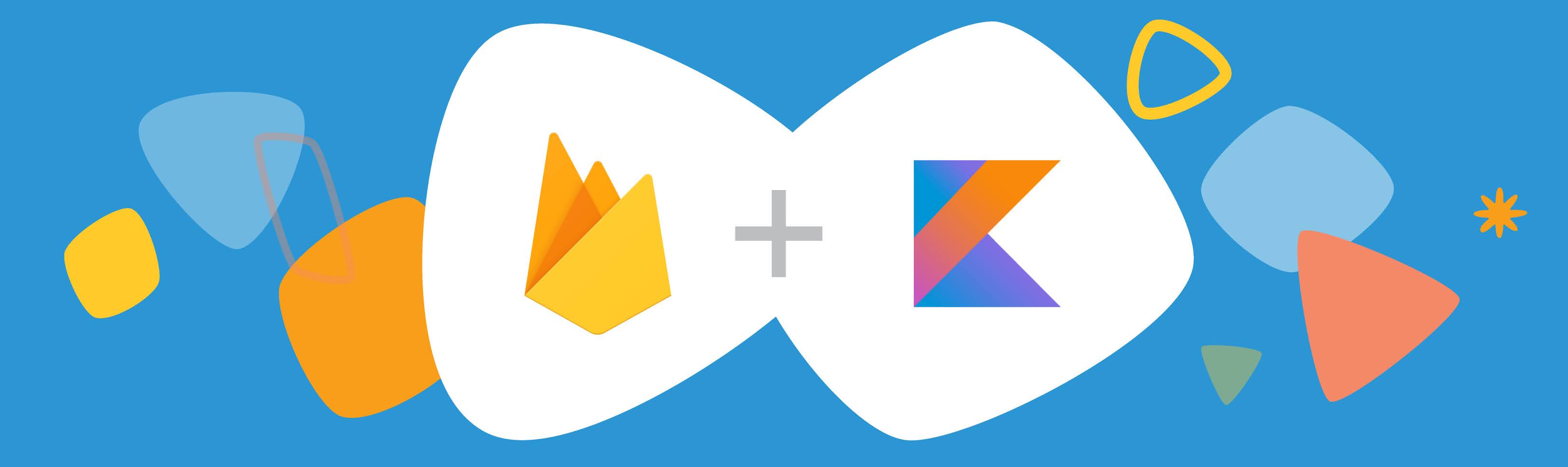
Last year we announced our investment in making Firebase libraries more Kotlin-friendly with Firebase Kotlin extension (KTX) libraries. Since then we have seen increasing interest for Kotlin within the Firebase community. In this blog post, we’ll go over how developing with Kotlin can lead to fewer crashes; and how you can monitor your app’s stability with Firebase Crashlytics once your app has been released.
Users expect to have a seamless experience every time they use your app. Crashes can cause churn and poor reviews, and quality issues are one of the main causes of early app deletion. Android apps built with Kotlin have 20% fewer crashes, which is one of the reasons that over 70% of the Top 1000 apps on the Play store have adopted Kotlin. Using Kotlin allows you to reduce the chances of getting null pointer exceptions, which are the #1 type of crashes on Google Play.
Furthermore Kotlin extension libraries let you write cleaner code by reducing boilerplate and making it easier to take advantage of advanced Kotlin language features even when using libraries originally written in Java.
In addition to our Firebase KTX libraries, you can also use the coroutines Kotlin extension libraries by Jetbrains to write safer async code with Kotlin and Firebase:
dependencies {
// Coroutines
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:1.3.9'
// Coroutines extensions for the Tasks API
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-play-services:1.3.9'
// Firestore Kotlin extensions
implementation 'com.google.firebase:firebase-firestore-ktx:22.0.2'
}
Consider this code to fetch a document from Firestore. This code uses the Firebase Android SDK and the Play services Tasks library, both of which are Java libraries:
fun getUser() {
val query = FirebaseFirestore.getInstance()
.collection("users").document("user123")
// query.get() returns a Task, we attach a callback to get the result
query.get().addOnSuccessListener { snap ->
val user = snap.toObject(User::class.java)
}
}
By using the Kotlin extension libraries above we can make this code simpler, safer, and easier to read:
suspend fun getUser() {
// Firebase.firestore is a convenient syntax from the firestore-ktx library
val query = Firebase.firestore
.collection("users").document("user123")
// The kotlinx-coroutines-play-services library allows us to "await" the
// result of a Task and avoid callbacks, which simplifies our control flow
val snap = query.get().await()
// The .toObject<T> function from the firestore-ktx library uses Kotlin's support
// for advanced generics to avoid the need to pass a Class object
val user = snap.toObject<User>()
}
Example provided by Firebase GDE Rosario Pereira Fernandes
Once you’ve developed and released your app, Firebase Crashlytics helps you improve and monitor your app stability. With Crashlytics you can track, prioritize and fix stability issues that erode app quality, in real-time. For instance, custom logs and keys in Crashlytics provides you with information on the specific state of your app leading up to a crash and gives context on why a crash occurred. With this level of in-depth insight, you can uncover the root causes of crashes more quickly before they affect a large number of your users.
For further analysis of your Crashlytics data and to segment your user data, you can also export all your crash data to BigQuery in real-time. For example, you can determine emerging crashes in new code, or see the top crash issues for the day to help you prioritize and fix them faster. You can also use our Data Studio template to easily visualize this data with custom dashboards. Data Studio dashboards are easy to collaborate on and share so your team can work more efficiently; even your team members who aren’t comfortable with SQL can easily work around BigQuery data sets.
These are just a few examples of the ways you can improve your app stability with Firebase, Kotlin Extensions and Crashlytics. It’s easy to get started with Crashlytics and Firebase Kotlin Extension libraries, and as always if you need help please feel free to reach out to us through our Community Slack.
Happy developing!