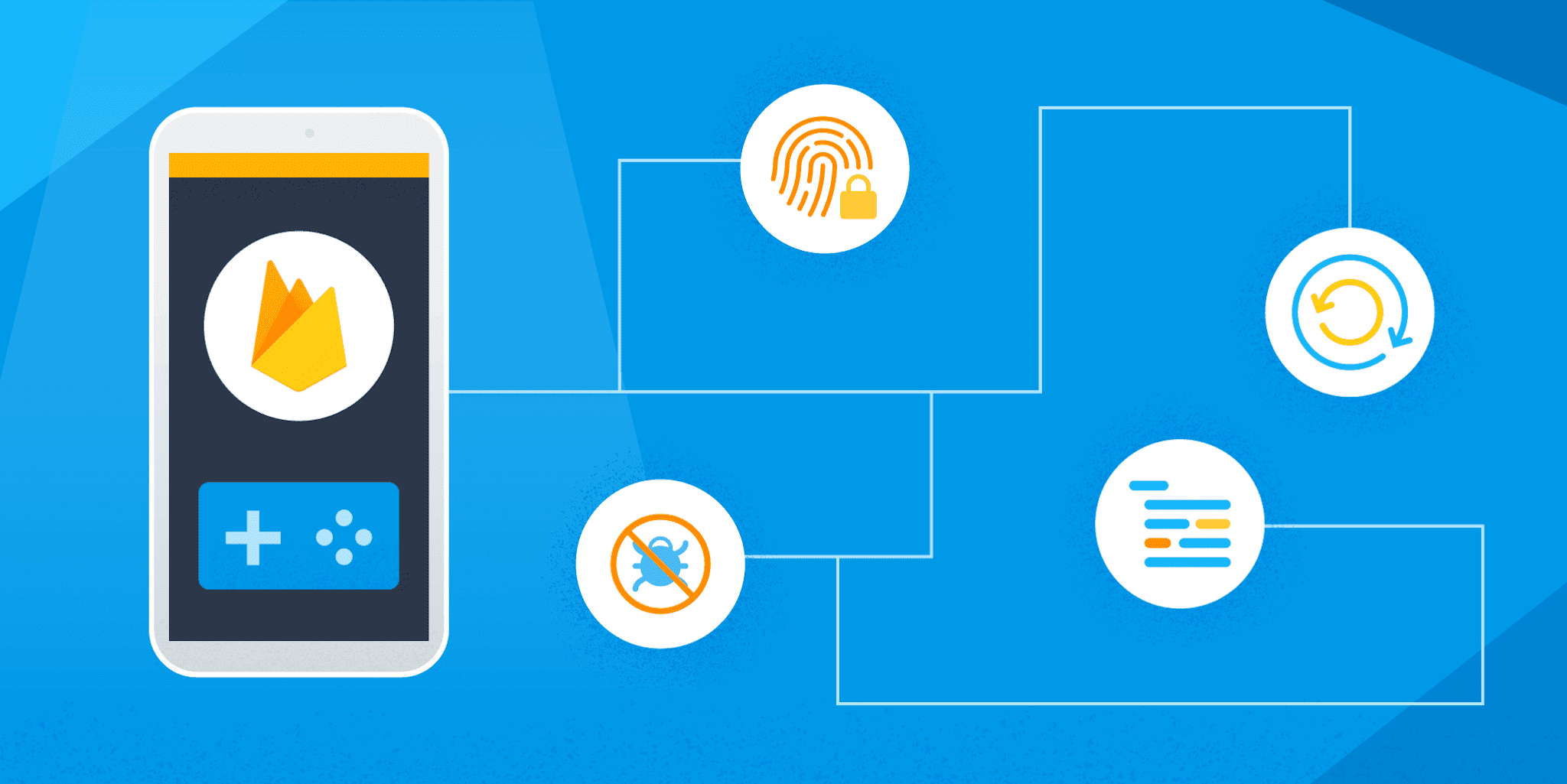
Version 7.1.0 of the Firebase Unity and C++ SDKs did a lot to improve Firebase Remote Config and better aligned it with the iOS and Android SDKs. In the process, however, there were some minor changes to the API that may require some action on your part.
Deprecation of the old API
First, in the Unity/C# SDK, the static methods you’ve been using have been removed from FirebaseRemoteConfig
and moved into FirebaseRemoteConfigDeprecated
. For technical reasons, we could not deprecate these functions and leave them in place. If you want a fast and easy way to update Firebase in your game, change FirebaseRemoteConfig
to FirebaseRemoteConfigDeprecated
. This will let your existing code work without any additional changes, but you won’t be able to take advantage of the newer features.
Also worth noting is that IsDeveloperMode
no longer has any effect and can be omitted. You likely paired this with a change to MinimumFetchInterfaclInMilliseconds
or by passing a TimeSpan
into FetchAsync
when debugging Remote Config. Shorter fetch intervals now function without IsDeveloperMode
making RemoteConfig an even stronger tool in your LiveOps toolbox!
C++ developers can leave their code as is, although you will be warned about deprecation.
New Features
Switch to instances
Developers wishing to future proof their code and take advantage of new features as they become available should switch to using a Remote Config instance rather than using static functions.
// Unity/C#
var remoteConfig = FirebaseRemoteConfig.DefaultInstance;
// C++
auto remoteConfig = ::firebase::remote_config::RemoteConfig::GetInstance(app);
SetDefaults
is now asynchronous.
SetDefaults
is now asynchronous. This is probably a good thing for game developers hoping to move logic off of the main thread, but you do have to be a little more cautious when building your app logic.
// Unity/C#
remoteConfig.SetDefaultsAsync(new Dictionary<string, object>
{
{"starting_player_lives", 3},
{"theme", "dark"}
}).ContinueWithOnMainThread(task =>
{
// it's now safe to Fetch
});
// C++
std::vector<remote_config::ConfigKeyValueVariant> defaultConfig = {
{"starting_player_lives", 3},
{"theme", "dark"},
};
remoteConfig->SetDefaults(defaultConfig.data(), defaultConfig.size())
.OnCompletion([](const ::firebase::Future<void>&){
// it's now safe to Fetch
});
Fetch and Activate in a single call
It’s now possible to Fetch and Activate Remote Config changes in a single call.
// Unity/C#
remoteConfig.FetchAndActivateAsync().ContinueWithOnMainThread(task =>
{
// handle completion
});
// C++
remoteConfig->FetchAndActivate().OnCompletion([](const ::firebase::Future<bool>& future) {
// handle completion
});
Retrieve values in native collection
And when you are ready to read your configuration, it’s now possible to retrieve all of the keys and values in a single language-specific native collection. No more getting a list of keys and looking up each value individually!
// Unity/C#
IDictionary<string, ConfigValue> values = remoteConfig.AllValues;
// C++
std::map<std::string, ::firebase::Variant> values = remoteConfig->GetAll();
Debug settings
Finally, if you would like to debug your Remote Config settings, you may want to reduce the interval between fetches.
// Unity/C#
var configSettings = new ConfigSettings();
if (Debug.isDebugBuild)
{
// refresh immediately when debugging
configSettings.MinimumFetchInternalInMilliseconds = 0;
}
FirebaseRemoteConfig.DefaultInstance.SetConfigSettingsAsync(configSettings).ContinueWithOnMainThread(task =>
{
// handle completion
});
// C++
::firebase::remote_config::ConfigSettings settings;
settings.minimum_fetch_interval_in_milliseconds = 0; // caution, only do this in debug mode!
remoteConfig->SetConfigSettings(settings);
More to Come!
These changes are part of an ongoing effort to improve Remote Config, including some exciting new features you’ll be hearing about in the future. Updating your games now will ensure that you can continue to take the advantage of the latest advances in Remote Config.