With Android App Bundles, you can separate your app into a base module and feature modules, allowing certain features of your app to be delivered conditionally or downloaded on demand. Each user of your app will receive only the code they need, when they need it.
As of the 28.0.0 release of the Firebase BoM, you can now use Firebase SDKs in feature modules of your app, not just your base module! This means you can reduce your app size if you’re only using Firebase services in some of your modules.
For instance, imagine you have an app which uses Firebase Authentication for login and has an optional “chat with support” feature which uses Firebase Realtime Database. In a monolithic app structure, all of these features would be included in the base app module and delivered at install time:
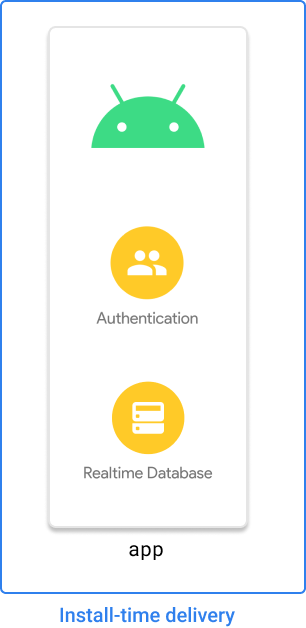
With the latest version of the Firebase Android SDK, you could use feature modules to separate each feature. The signin feature would still be delivered at install time, since most users need to sign in. However, by moving the “chat with support” module to a feature module configured with on-demand delivery, it would be delivered only when the user needs it:
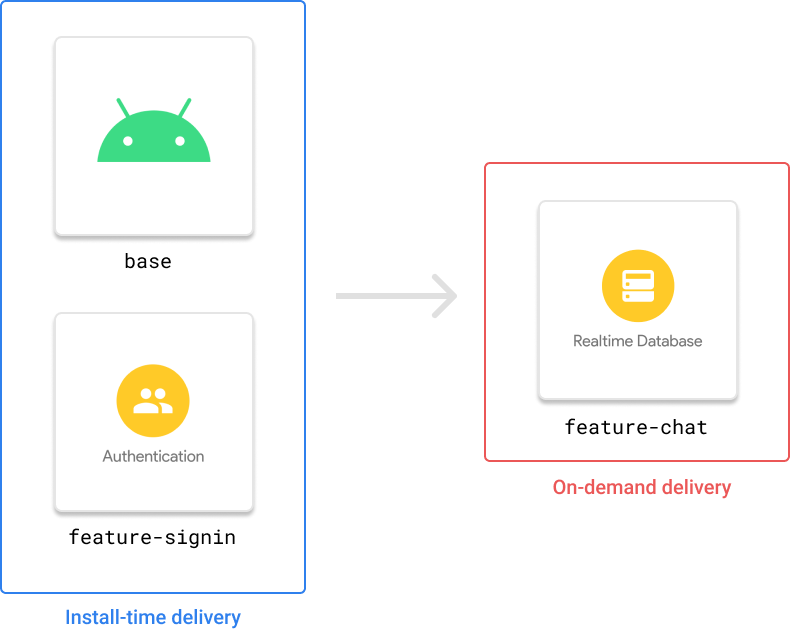
With this modular structure, your app’s initial download size is much smaller. The feature module will defer downloading of not only the Realtime Database SDK, which is about 400kB, but also all the code, strings, resources, and assets needed to build the chat module.
To get started, just add the following dependency to your app’s base module:
// base module
dependencies {
// Note: this library is not yet included in the Firebase BoM
implementation 'com.google.firebase:firebase-dynamic-module-support:16.0.0-beta01'
}
Then, you can add Firebase dependencies to feature modules and use them as you normally would.
// feature-signin module
dependencies {
implementation platform('com.google.firebase:firebase-bom:28.0.0')
implementation 'com.google.firebase:firebase-auth'
}
// feature-chat module
dependencies {
implementation platform('com.google.firebase:firebase-bom:28.0.0')
implementation 'com.google.firebase:firebase-database'
}
By using a modular architecture, you can reduce the initial download size of your app and progressively enhance it with rich, Firebase-powered features as needed.
To read more about feature modules and how they work with the Firebase Android SDK, check out the documentation: