Developers! Firebase Cloud Messaging now supports notifications on Safari. Thanks to Apple’s support for the Push API from Safari 16.1 and macOS 16.4 iOS & iPadOS, you can now send Firebase Cloud Messaging notifications to your web applications served on these versions of Safari. This opens up new possibilities for engaging with your users and enhancing the user experience and complements the wide range of existing platforms supported such as Chrome and Edge browsers
In this blog post, we’ll provide an overview of how to enable web push notifications for Safari using Firebase Cloud Messaging. We’ll cover everything you need to know including code snippets for critical setup and configuration.
By following these steps, you’ll be able to send push notifications to your users on Safari and enhance their experience with your app. Let’s dive in and explore how to take advantage of this new feature!
How to Enable Firebase Cloud Messaging on Safari for macOS
Enabling Firebase Cloud Messaging for Safari on macOS requires just a few steps. To get started, we recommend checking out the Firebase FCM JS SDK quick start guide on GitHub. It’s an out-of-the-box sample app that works on most browsers including Safari. This guide provides a detailed walkthrough for setting up Firebase Cloud Messaging in your web app and a code sample that you can use as a starting point.
To enable the Push API for Safari, make sure to wrap the requestPermission()
API inside a user action, such as a button click.
By enabling web push notifications for Safari, you’ll be able to increase engagement with your users, improve the user experience, and increase visibility and awareness for your app. And that’s it!
How to Enable Firebase Cloud Messaging on Safari for iOS and iPadOS
Enabling Firebase Cloud Messaging for Safari on iOS and iPadOS requires some additional steps beyond those required for macOS.
Configure the manifest.json file
Configure your web application to set “display”: “standalone” or “fullscreen” in the manifest.json file, which allows your app to be added to the Home Screen.
{ "display": "standalone" } // or {"display": "fullscreen"}
Add to home screen
Prompt users to add your app to the home screen. It’s a requirement to add the app to the home screen for push notifications. Once users install your app on the home screen, it will receive push notifications.
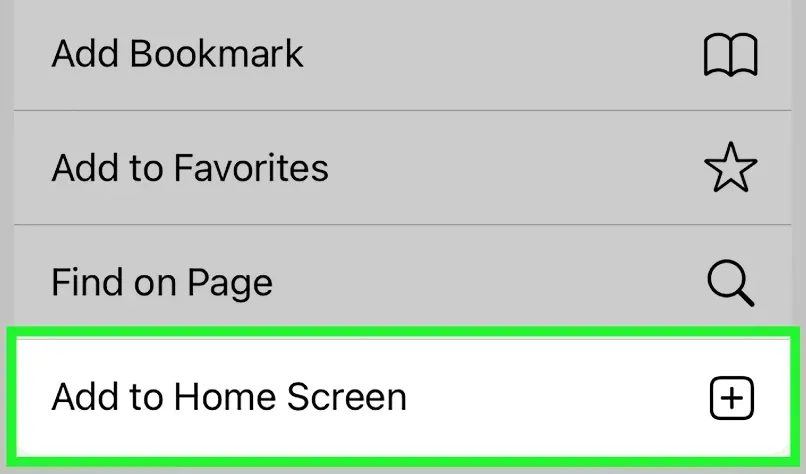
Request push notification permissions
Request permission to receive push notifications in response to direct user interaction, such as tapping on a ‘subscribe’ button provided by the web app.
let permission = await Notification.requestPermission();
if (permission === "granted") {
// Get the FCM token (see below)
} else {
// Handle denied permission
}
Initialize FCM
Now that we have the user’s permission let’s initialize Firebase Cloud Messaging by adding the following code snippet to your JavaScript:
// Example: wrapping permission request call inside a button click
const myButton = document.querySelector("button");
myButton.addEventListener("click", async () => {
let permission = await Notification.requestPermission();
if (permission === "granted") {
console.log("Notification permission granted. Requesting for token.");
let token = await messaging.getToken({
vapidKey: "<YOUR_PUBLIC_VAPID_KEY_HERE>",
});
// do something with the FCM token
} else {
console.log("Notification permission denied");
// Handle denied permission
}
});
Sending notifications
Now you can send a notification to the application either through v1 Send API (below we show a code snippet) or the Firebase console.
{
"message": {
"token": "bk3RNwTe3H0:CI2k_HHwgIpoDKCIZvvDMExUdFQ3P1...",
"notification": {
"body": "This is an FCM notification message!",
"title": "FCM Message"
}
}
}
To learn more about sending through the Firebase console, please refer to https://firebase.google.com/docs/cloud-messaging/js/send-with-console
By following these steps, you can enable web push notifications for Safari on iOS and iPadOS using Firebase Cloud Messaging.
APNs & FCM
It’s important to note that web push on iOS and iPadOS uses the same Apple Push Notification service that powers native push on all Apple devices. You don’t need to be a member of the Apple Developer Program to use it, but be sure to allow URLs from *.push.apple.com if you control your server push endpoints.
Conclusion
Enabling web push notifications for Safari on both macOS and iOS/iPadOS using Firebase Cloud Messaging is now possible with recent updates from Apple adding support for the Push API in Safari. With Firebase Cloud Messaging, you can increase engagement, enhance user experience, and increase the visibility for your app. Follow the steps outlined in this post and reference the Firebase FCM JS SDK quick start guide on GitHub to get started. Contact the Firebase community if you need help or have feedback. We’re here to help you on your development journey.