With Firebase Data Connect’s recent public preview launch, we’re excited to announce the launch of one of the most requested features: native Flutter SDKs for Data Connect! In this post, we’ll go over how to use them in your app.
What is Firebase Data Connect?
At Google I/O, we launched Firebase Data Connect, a backend-as-a-service powered by a Cloud SQL Postgres database that’s high performance, scalable, and secure. It utilizes GraphQL to provide users a way to define your data and the relationships between them, as well as interactions with your data.
Our existing offering provided Android, iOS, and Web support, and now we’re excited to announce a new set of SDKs for Flutter, written entirely in native Dart. With a new generated SDK, in a few lines of Flutter code, you get access to your Cloud SQL data with full type-safety included!
Instead of having to type out parseJsonToMyMovie(myOrm().select('title, genre, description').from('movies'));
, all you have to do is call MoviesConnector.instance.listMovies();
and you’re good to go!
Can’t wait to try it out? Install our VSCode extension and follow the steps below, or take a look at the documentation.
Getting started
If you haven’t already, add Firebase to your Flutter app by following these steps.
To start generating the new Flutter SDK, add the following configuration to your dataconnect/connector/connector.yaml
file:
generate:
dartSdk:
outputDir: "./lib/generated"
package: "movies"
The outputDir
specifies where the generated SDKs should live, and the package
defines the name of the new Dart package where the generated code will live.
Next, let’s add some tables to our schema:
type Movie @table {
# The below parameter values are generated
# by default with @table, and can be edited
# manually.
#
# implies directive '@col(name: "movie_id")',
# generating a column name
id: UUID! @default(expr: "uuidV4()")
title: String!
imageUrl: String!
genre: String
}
Add a new query to our list of operations:
# @auth() directives control who can call
# each operation.
# Anyone should be able to list all movies,
# so the auth level is set to PUBLIC
query ListMovies @auth(level: PUBLIC) {
movies {
id
title
imageUrl
genre
}
}
Note: We use PUBLIC
in the example below as this doesn’t expose sensitive information. We recommend using stricter auth on restrictive operations.
And add a new mutation to add a new movie into the list:
mutation CreateMovie(
$title: String!
$genre: String!
$imageUrl: String!
) @auth(level: USER) {
movie_insert(
data: {
title: $title
genre: $genre
imageUrl: $imageUrl
}
)
}
Now let’s call it!
First, install the Data Connect core SDKs:
Then, add the following to your main.dart
:
...
import 'package:fdc_flutter_blog_post/generated/movies.dart';
...
// Add logic to log in a user
...
// Add a movie if one doesn't exist already
await MoviesConnector.instance
.createMovie(
title: "Quantum Paradox",
genre: "sci--fi",
imageUrl:
"https://firebasestorage.googleapis.com/v0/b/fdc-web-quickstart.appspot.com/o/movie%2Fquantum_paradox.jpeg?alt=media&token=4142e2a1-bf43-43b5-b7cf-6616be3fd4e3")
.execute();
// Call the ListMovies query we created earlier
MoviesConnector.instance.listMovies().execute().then((res) {
setState(() {
_movies = res.data.movies;
});
});
Here’s a sample app using that query:
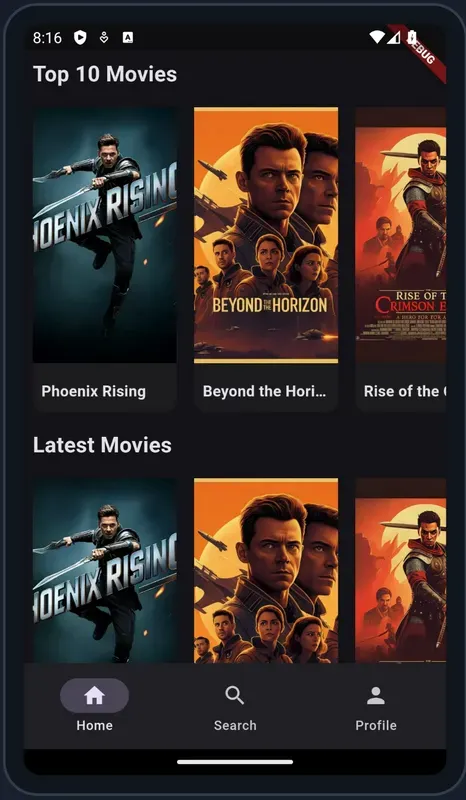
Learn More!
To learn more about developing Flutter apps using Data Connect, visit our documentation, and try out our quickstart by cloning our GitHub repo!