Just in time for EmberConf next week, we’re excited to announce a new version of our EmberFire bindings, along with an example blogging app to showcase the magic of using Firebase with Ember.
Our new Ember bindings now work directly with Ember Data. This means you can now use Ember Data’s relationships with Firebase to define hasMany and belongsTo relationships between your models. If you’re new to Ember Data, Ember’s guide to models is a great place to start.
With these updates, EmberFire now works only with Ember Data. For Ember developers who aren’t using Ember Data, Michael Jackson has written ember-firebase - an awesome, thoroughly tested set of Firebase bindings for Ember. If you’d still like to use the previous version of EmberFire, it is available in the v0.1.0 release on GitHub.
Getting Started With the New EmberFire
With our new bindings, you can start using EmberFire in just two steps. First, you’ll need to include the necessary libraries:
<head>
<!-- Ember + Ember Data -->
<script src="http://builds.emberjs.com/canary/ember.min.js"></script>
<script src="http://builds.emberjs.com/canary/ember-data.min.js"></script>
<!-- Firebase -->
<script src="https://cdn.firebase.com/js/client/1.0.17/firebase.js"></script>
<!-- EmberFire -->
<script src="https://cdn.firebase.com/libs/emberfire/1.0.13/emberfire.min.js"></script>
</head>
You can also download EmberFire and all its dependencies from Bower via bower install emberfire --save
.
Then, simply create an instance of the DS.FirebaseAdapter
in your app and add your Firebase URL:
App.ApplicationAdapter = DS.FirebaseAdapter.extend({
firebase: new Firebase('https://<my-firebase>.firebaseio.com')
});
With the adapter set up, you can now interact with the data store as you normally would with Ember. For example, calling find() with a specific ID will retrieve that record from Firebase. It will also start watching for updates and will update the data store automatically whenever anything is added or removed.
FireBlog
To help you understand our new EmberFire bindings we’ve created FireBlog, a realtime blogging app with posts, comments, and users. We’ll walk through the core concepts here, and the full code is available on GitHub.
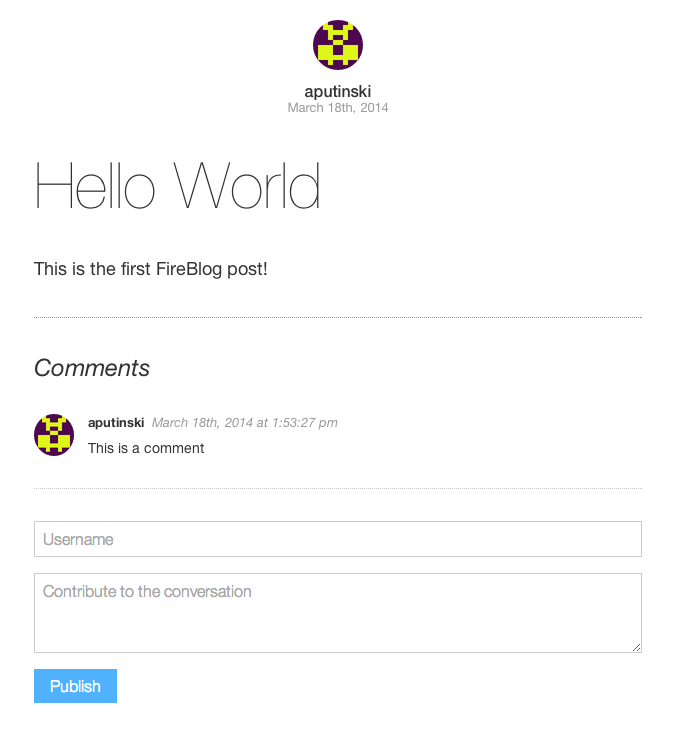
Structuring Data in EmberFire
Because both Ember Data and Firebase work best with denormalized data, FireBlog does not embed comment data directly in each post. Instead, each post has a comments object with a comment ID pointing to the comment data in /comments
. We’ve created three top-level keys for posts, comments, and users, and the data structure in Firebase looks like this:
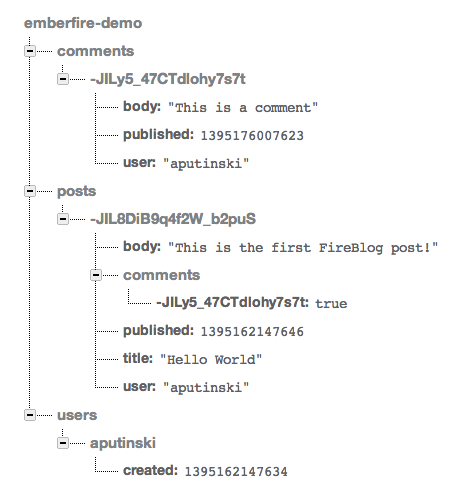
Setting Up a Model
Each post in our blog will have a title, body, published, user, and comments attributes. Using Ember Data’s relationships, each post belongsTo a user and hasMany comments. We’ll use Ember’s computed properties to format the date to display on the blog post.
App.Post = DS.Model.extend({
title: DS.attr('string'),
body: DS.attr('string'),
published: DS.attr('number'),
publishedDate: function() {
return moment(this.get('published')).format('MMMM Do, YYYY');
}.property('published'),
user: DS.belongsTo('user', { async: true }),
comments: DS.hasMany('comment', { async: true })
});
The comment and user models are set up in a similar way, which you can see on GitHub.
Fetching All Posts
To get all blog posts from Firebase we can use the standard Ember Data way of fetching posts, like the following:
App.PostsIndexRoute = Ember.Route.extend({
model: function() {
return this.store.findAll('post');
}
});
This will fetch all posts when the page first loads, and will also listen for updates to Firebase. Whenever posts are added, the data store will update automatically and new posts will be displayed in the DOM.
Displaying a Single Post
Similar to how we fetched all posts using store.findAll()
, we’ll fetch individual posts using store.find()
:
App.PostRoute = Ember.Route.extend({
model: function(params) {
return this.store.find('post', params.post_id);
}
});
We’ll use the following template to display a single post. Then we’ll create an Ember.Component
to display individual blog posts. We’ll pass in the model from the route (post=model) as a property to show the post’s title, author, date, and comments:
<script type="text/x-handlebars" data-template-name="post">
{{ "{"}}{{ "{"}}fire-post post=model onPublishComment="publishComment"}}
</script>
Here is the App.FirePostComponent
we use to display an individual blog post:
<script type="text/x-handlebars" data-template-name="components/fire-post">
<div class="post-header">
<img {{ "{"}}{{ "{"}}bind-attr src="post.user.avatar"}} class="avatar post-avatar"/>
<div class="post-author">{{ "{"}}{{ "{"}}post.user.id}}
<div class="post-date">{{ "{"}}{{ "{"}}post.publishedDate}}
<h3 class="post-title">{{ "{"}}{{ "{"}}post.title}}</h3>
<div class="post-content">{{ "{"}}{{ "{"}}breaklines post.body}}
<ul class="post-comments">
{{ "{"}}{{ "{"}}#each comment in post.comments}}
<li class="post-comment">
<img {{ "{"}}{{ "{"}}bind-attr src="comment.user.avatar"}} class="avatar post-comment-avatar" />
<div class="post-comment-body">
<div class="post-comment-meta">
<strong class="post-comment-author">{{ "{"}}{{ "{"}}comment.user.username}}</strong>
<em class="post-comment-date">{{ "{"}}{{ "{"}}comment.publishedDate}}</em>
{{ "{"}}{{ "{"}}breaklines comment.body}}
</li>
{{ "{"}}{{ "{"}}/each}}
</ul>
</script>
Now we have a full-featured blogging application that displays posts and comments in realtime without a server. It’s that simple!
Send Us Your Feedback
Our goal with the new EmberFire library is to make the process of adding a backend to your Ember app and integrating with Ember Data as seamless as possible. We encourage you to take our new bindings for a spin. Submit a pull request or email me at [adam@firebase.com](mailto:adam@firebase.com?subject=EmberFire Feedback) with any feedback. We’re just getting started with this new Ember Data integration, and we’re working hard to make updates based on your suggestions. We’re excited to see what you build with Firebase and Ember.
For those of you going to EmberConf, look for James, Alex, Sara and I in the bright yellow t-shirts!