Today we’re excited to announce Firebase Hosting integration for Google Cloud’s new Cloud Run service. Cloud Run is a fully managed compute platform that enables developers to run stateless containers that are invocable via HTTP requests in a language and framework of their choosing. Firebase Hosting integration lets you use this architecture as a backend for a web app or microservice in your Firebase project.
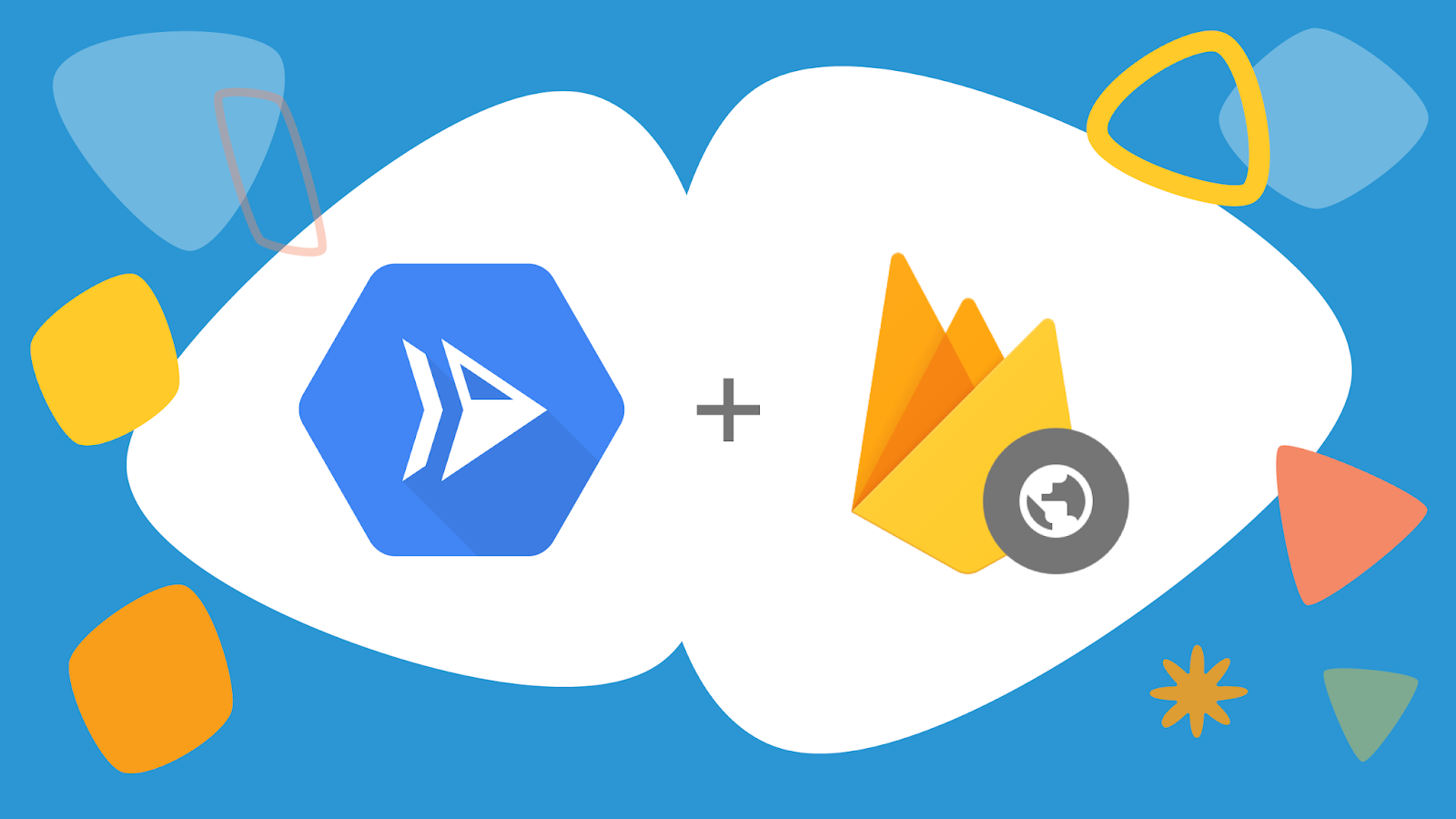
Microservices on Firebase Hosting
Firebase Hosting is already a convenient and secure way to host sites and microservices. It can serve static pages you upload directly and, with the proper configuration in the firebase.json file, direct incoming requests to Cloud Functions for Firebase to serve dynamic content. This workflow is a one stop shop if you don’t mind working in the Node.js environment. You can already build a fast site with dynamic content that automatically scales horizontally to meet user demand.
Not every developer wants to work with Node.js though. Many already have large teams with existing knowledge in other languages and frameworks. Languages such as Go, Ruby, and Java have a huge presence in the server market but are currently absent in Firebase’s existing cloud backend solutions.
Deploy containers to GCP, rewrite through Firebase Hosting
Leveraging the power of Google’s own experience building infrastructure for Kubernetes and the efforts of the Knative open source project, Google Cloud Platform now lets you deploy stateless servers. The only requirements are that you can generate a docker image able to interact to HTTP requests on the port specified in the $PORT environment variable for Kubernetes and that you respond within 60 seconds for Firebase Hosting. How does this tie into Firebase Hosting though?
Setting up rewrite configuration
If you’re new to Hosting, you may only be aware of static hosting or the free SSL certificates. To facilitate serving dynamic content, rewrites allow you to hit your cloud functions, which we’ve extended to support Cloud Run as well. With a few minor changes to your firebase.json file, you can now point a specific path to your container:
{
"hosting": {
"public": "public",
"rewrites": [ {
"source": "/cloudrun",
"run": {
"serviceId": "my-awesome-api",
// Optional (default is us-central1)
"region": "us-central1",
}
} ]
}
}
or use wildcards to expose an entire API
{
"hosting": {
"public": "public",
"rewrites": [ {
"source": "/api/**",
"run": {
"serviceId": "my-awesome-api",
// Optional (default is us-central1)
"region": "us-central1",
}
} ]
}
}
Managing the CDN cache
If you have a dynamic site that doesn’t update very frequently, take advantage of Firebase Hosting’s global CDN (content delivery network) to improve your site’s response time. For example, if you’re using ExpressJS and Node.js, configure the caching behavior using the Cache-Control
header like so:
res.set('Cache-Control', 'public, max-age=300, s-maxage=600');
This caches the results of a request in the browser (max-age
) for 5 minutes and in the CDN (s-maxage
) for 10 minutes. With properly tuned cache settings, you can have a fast, flexible, dynamically rendered site that doesn’t need to run your server logic every time the user opens the page.
Cloud Run features
Use any server language
Unlike Cloud Functions for Firebase, when you use Cloud Run, you can build an image with any combination of languages and frameworks to handle these requests. Ruby developers can easily pull in Sinatra, you can fire up the Spring framework for Java teams, or check out server side Dart using Shelf to serve content. You don’t have to wait for any official language support — if you can create a docker container, you can make and deploy backend code. Even if you’re working in high performance computing and your engineering team is trained up in Fortran, you can just leverage that existing knowledge to create a web dashboard with Fortran.io without having to wait for any official support from Google or Firebase.
Auto-scale horizontally
Similar to Cloud Functions, Cloud Run automatically scales your containers horizontally to meet the demands of your users. There’s no need to manage clusters or node pools; you simply use the resources needed at the time to accomplish the task at hand.
Concurrency
One tradeoff is that, also like Cloud Functions, Cloud Run images are stateless. However, unlike Cloud Functions, each container can handle up to 80 concurrent users, which can help reduce the frequency of cold starts.
Learn more
Using Firebase Hosting with Cloud Run, we hope to empower you to build better web apps faster than ever before. Now your frontend and backend developers can truly use a single language, even share a code base. To get started right away, follow our step-by-step guide. Note that Cloud Run exists in the Google Cloud console rather than the Firebase console, but if you have a Firebase project then you already have a Google Cloud Platform project as well.