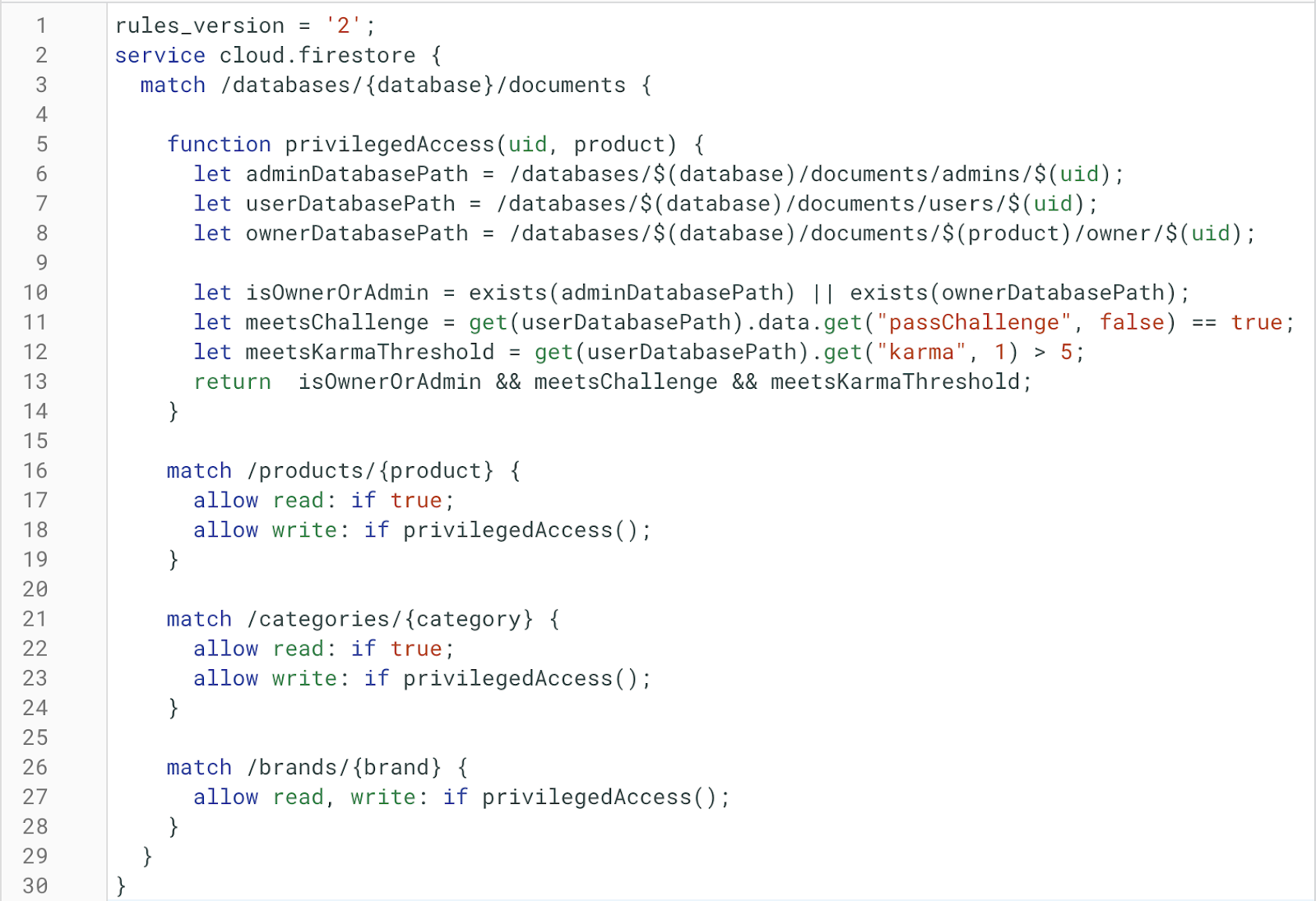
If you’re using Cloud Firestore or Cloud Storage for Firebase, you’re also using Security Rules. (If you’re using the default rules instead of tailoring them to your app, this is where to start!) We’re excited to announce that in the last few months we’ve released some substantial improvements to the tools for writing and debugging Rules, improvements to the Rules language itself, and increases to the size limits for Rules!. These are a few of the great new features. Check out the Security Rules Release Notes for a comprehensive list of everything we’ve released.
Sets
We’ve released several improvements to make the rules language more expressive and succinct. One particularly verbose pattern was comparing the new values of a document to existing values. The new [Set type](https://firebase.google.com/docs/reference/rules/rules.Set)
available in Rules is purpose-built for these comparisons, and also has methods for functionality you’d expect for a Set, like getting the intersection, union, or difference between Sets. For example:
Allow a user to create a document if the document has required and optional fields, but not others:
allow create: if (request.resource.data.keys().toSet()
.hasOnly(["required","and","optional","keys"])
Sets come with ==
and in
operators and hasAll
, hasAny
, hasOnly
, difference
, intersection
, union
, and size
methods.
New Map methods
Sets are most useful in conjunction with the [Map](https://firebase.google.com/docs/reference/rules/rules.Map)
class, and because the request
and resource
objects are both structured as maps, you’re probably already familiar with it. Map
recently got a few new methods, diff
and get
, that will hopefully open the door to more concise rules for everyone. Here’s how they work:
Map.diff() is called on one map, and takes the second map as an argument: map1.diff(map2)
. It returns a MapDiff object, and all of the MapDiff methods, like addedKeys
, changedKeys
, or affectedKeys
return a Set
object.
Map.diff() can solve some verbose patterns like checking which fields changed before and after a request. For example, this rule allows an update if the “maxLevel” field was the only field changed:
allow update: if
request.resource.data.diff(resource.data).changedKeys().hasOnly(["maxLevel"]);
In the next example, posts have a field indicating the user role required to modify the post. We’ll use Map.get() to get the "roleToEdit"
field. If the document doesn’t have the field, it will default to the "admin"
role. Then we’ll compare that to the role that’s on the user’s custom claims:
allow update, delete: if resource.data.get("roleToEdit", "admin") == request.auth.token.role;
Keep in mind that because Sets are not ordered but Lists are. You can convert a List to a Set, but you can’t convert a Set to a List.
Local variables
Local variables have been one of the most requested features in Rules, and they’re now available within functions. You can declare a variable using the keyword let
, and you can have up to 10 local variables per function.
Say you’re commonly checking that a user meets the same three conditions before granting access: that they’re an owner of the product or an admin user, that they successfully answered a challenge question, and that they meet the karma threshold.
rules_version = '2';
service cloud.firestore {
match /databases/{database}/documents {
match /products/{product} {
allow read: if true;
allow write: if (exists(/databases/$(database)/documents/admins/$(request.auth.uid))
|| exists(/databases/$(database)/documents/product/owner/$(request.auth.uid)))
&& get(/databases/$(database)/documents/users/$(request.auth.uid))
.data.passChallenge == true
&& get(/databases/$(database)/documents/users/$(request.auth.uid))
.data.karma > 5;
}
match /categories/{category} {
allow read: if true;
allow write: if (exists(/databases/$(database)/documents/admins/$(request.auth.uid))
|| exists(/databases/$(database)/documents/product/owner/$(request.auth.uid)))
&& get(/databases/$(database)/documents/users/$(request.auth.uid))
.data.passChallenge == true
&& get(/databases/$(database)/documents/users/$(request.auth.uid))
.data.karma > 5;
}
match /brands/{brand} {
allow read, write: if (exists(/databases/$(database)/documents/admins/$(request.auth.uid))
|| exists(/databases/$(database)/documents/product/owner/$(request.auth.uid)))
&& get(/databases/$(database)/documents/users/$(request.auth.uid))
.data.passChallenge == true
&& get(/databases/$(database)/documents/users/$(request.auth.uid))
.data.karma > 5;
}
}
}
Those conditions, along with the paths I’m using for lookups can all now become variables in a function, which creates more readable rules:
rules_version = '2';
service cloud.firestore {
match /databases/{database}/documents {
function privilegedAccess(uid, product) {
let adminDatabasePath = /databases/$(database)/documents/admins/$(uid);
let userDatabasePath = /databases/$(database)/documents/users/$(uid);
let ownerDatabasePath = /databases/$(database)/documents/$(product)/owner/$(uid);
let isOwnerOrAdmin = exists(adminDatabasePath) || exists(ownerDatabasePath);
let meetsChallenge = get(userDatabasePath).data.get("passChallenge", false) == true;
let meetsKarmaThreshold = get(userDatabasePath).data.get("karma", 1) > 5;
return isOwnerOrAdmin && meetsChallenge && meetsKarmaThreshold;
}
match /products/{product} {
allow read: if true;
allow write: if privilegedAccess();
}
match /categories/{category} {
allow read: if true;
allow write: if privilegedAccess();
}
match /brands/{brand} {
allow read, write: if privilegedAccess();
}
}
}
You can see at a glance that the same conditions grant access to write to documents in the three different collections.
The updated version also uses [map.get()](https://firebase.google.com/docs/reference/rules/rules.Map#get)
to fetch the karma
and passChallenge
fields from the user data, which helps keep the new function concise. In this example, if there is no karma
field for a user, then the get
returns false. Keep in mind that Map.get()
fetches a specific field, and is separate from the [DocumentReference.get()](https://firebase.google.com/docs/reference/js/firebase.firestore.DocumentReference#get)
that fetches a document.
Ternary operator
This is the first time we’ve introduced an if/else
control flow, and we hope it will make rules smoother and more powerful.
Here’s an example of using a ternary operator to specify complex conditions for a write. A user can update a document in two cases: first, if they’re an admin user, they need to either set the field overrideReason
or approvedBy
. Second, if they’re not an admin user, then the update must include all the required fields:
allow update: if isAdminUser(request.auth.uid) ?
request.resource.data.keys().toSet().hasAny(["overrideReason", "approvedBy"]) :
request.resource.data.keys().toSet().hasAll(["all", "the", "required", "fields"])
It was possible to express this before the ternary, but this is a much more concise expression.
The size limit for rules files is now 256 KB!
And finally, here’s a feature for those of you with longer rules. Until now, rules files had to be smaller than 64 KB. (To be more specific, the compiled AST of the rules file had to be smaller than 64 KB, and you wouldn’t know you were within the limit until you tried to deploy the rules.) This limit was holding some developers back, and once you reached the limit, you had to start making tradeoffs in your rules. We definitely wanted to fix this.
Since this is one of the limits that helps rules return a decision in nanoseconds, we wanted to find a way to increase the limit without sacrificing performance. We optimized how we compile and store the Rules file, and we were able to quadruple the limit to 256 KB!
The limits on rules are in place to keep rules fast enough to return a decision in nanoseconds, but we work hard to keep them workable. Let us know if you start to outgrow any of them
All of these features are informed by the feedback we hear from you about what’s great, what’s hard, and what’s confusing about Firestore Security Rules, so keep letting us know what you think!