Firebase introduced a new Google Mobile Ads C++ SDK in June 2022 to help you more easily monetize your app or game. This new SDK replaces the now deprecated AdMob C++ SDK offering, updating the API with modern C++ best practices and simplifying its use. Our goal was to streamline the process of adding revenue-generating banner ads, interstitial ads, and - most importantly - rewarded video ads to apps and games written in C++. Let’s take a look!
Note: if you’re currently using the deprecated Firebase AdMob C++ SDK and you’d like a clear path to implement these new features, please consult our handy Migration Guide.
Consistency of Use
The Google Mobile Ads C++ SDK consists of an API surface which wraps the iOS and Android Google Mobile Ads SDKs on those respective platforms. The C++ SDK normalizes the use and behaviors of the iOS and Android SDKs so that your logic to drive ad loads, displays, and track impressions can be written only once and it will run identically on both platforms.
Additionally, we’ve worked to normalize implementations for interstitial and rewarded full screen ad formats. Both are now implemented as classes (previously rewarded video ads were functions in a collective namespace). Ads now have class destructors that automatically clean-up the ad data in the underlying mobile SDKs.
Finally, interstitial ads and rewarded ads now share a common class interface for their content listeners. This should simplify how your app reacts to ad interactions and display events for both of these full screen ad types.
Stability First
The iOS and Android implementations of the Google Mobile Ads SDK require that apps invoke all operations on the main thread. We realize this could be difficult in C++, especially in call-back heavy game engines where it’s hard to funnel operations into a single thread. The new Google Mobile Ads C++ SDK handles this complexity for you, internally plumbing operations into a queue executed on the main thread. This simplifies usage, prevents potential misuse, and reduces the number of difficult-to-debug crashes, which harm overall user experience.
Insights into ad request fulfillment
Ad loads now return a Future containing an AdResult object for all ad view, interstitial ad and rewarded ad types. The AdResult::is_successful()
method returns true if the ad request was successfully fulfilled, or false if not.
On failure, the ad result contains an error object with service-level information about the problem, including the error code, the error message and domain strings, as well as information about why each adapter failed to fulfill the ad request. This information can help you troubleshoot network connectivity problems or mediation configuration issues.
On success, the ad result contains metrics about how long the request took, and which mediation adapter loaded the ad. These insights should provide you with information about why you’re seeing particular ad fulfillments, the performance of specific mediation adapters, and generally help you track where your ads are coming from.
Before
firebase::Future<AdResult> future;
void load_ad() {
// Assume an already created AdRequest and BannerView objects.
future = banner_view->LoadAd(ad_request);
}
void your_game_loop() {
firebase::Future<AdResult> future = ad_view->LoadAdLastResult();
if (future.status() == firebase::kFutureStatusComplete) {
if(future.error() != firebase::admob::kAdMobErrorNone) {
// There was either an internal SDK issue that caused the Future to
// fail its completion, or AdMob failed to fulfill the ad request.
// Details are unknown other than the Future’s error code returned
// from future.error().
} else {
// The ad loaded successfully but with little insight as to result.
}
}
}
After
firebase::gma::AdView* ad_view;
void load_ad() {
// Assumes a previously created AdRequest and AdView objects.
// See "AdRequest and Global Configuration" above.
ad_view->LoadAd(ad_request);
}
void your_game_loop() {
// Check the future status in your game loop:
firebase::Future<AdResult> future = ad_view->LoadAdLastResult();
if (future.status() == firebase::kFutureStatusComplete) {
if(future.error() != firebase::admob::kAdErrorCodeNone) {
// There was an internal SDK issue that caused the Future to fail.
} else {
// Future completed successfully. Check the GMA result.
const AdResult* ad_result = future.result();
if ( ad_result->is_successful() != true ) {
// GMA failed to serve an ad. Gather information about the error.
const AdError& ad_error = ad_result->ad_error();
AdErrorCode error_code = ad_error.code();
const std::string error_domain = ad_error.domain();
const std::string error_message = ad_error.message();
} else {
// The ad loaded successfully. Gather more information about the ad
// fulfillment.
std::string response_id = result_ptr->response_info().response_id();
std::string mediation_adapter_name =
result_ptr->response_info().mediation_adapter_class_name()
const std::vector<AdapterResponseInfo> adapter_responses =
ad_result->response_info().adapter_responses();
// Iterate over the adapter responses. Responses include latency information and
// why any particular adapter encountered errors attempting
// to fulfill the ad request (even if another adapter eventually fulfilled it
// successfully).
for( … ) { … }
}
}
}
}
Reward Your Users
The new Google Mobile Ads C++ SDK includes support for banner ads and interstitial ads which can be used to monetize your app. But when it comes to games, it’s rewarded video ads that provide a new level of engagement.
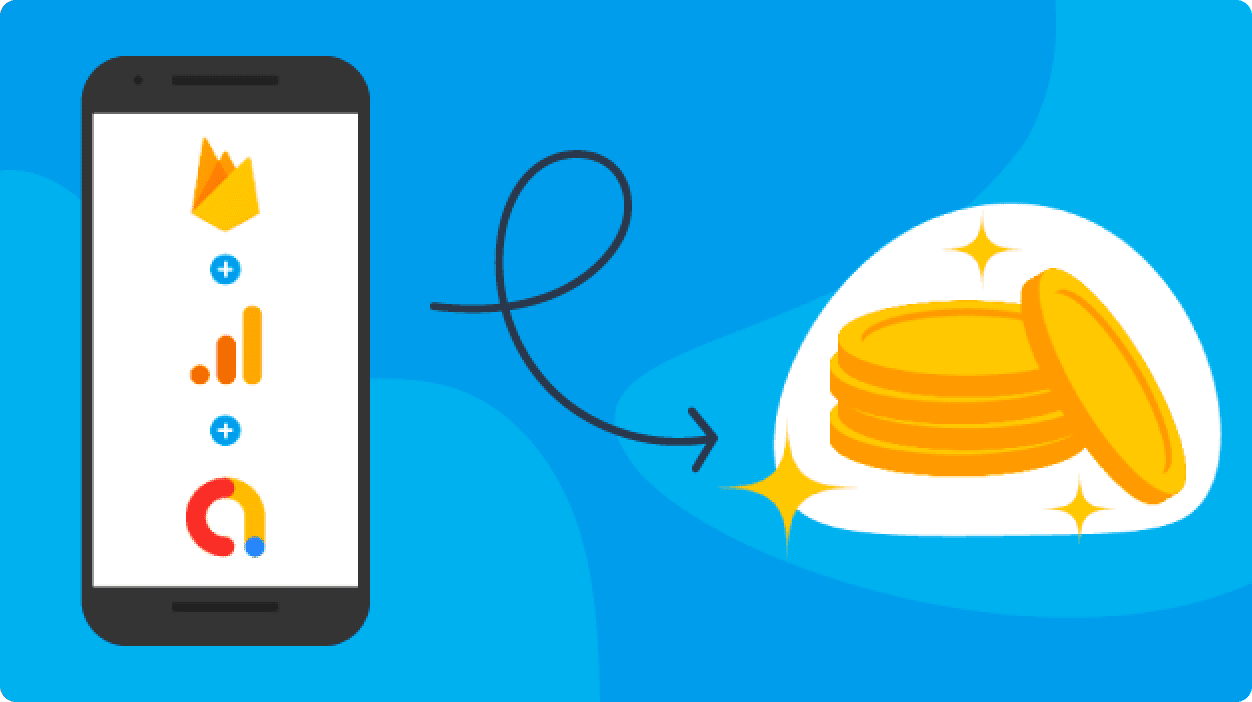
When you use a rewarded video ad, the Google Mobile Ads SDK will provide your application with an ad reward object after the user finishes watching an ad. This gives your app a hook so that you can reward the user with in-game items, currency, and unlocks.
And with server-side verification (SSV) callbacks, you can guarantee that a rogue app isn’t spoofing your backend systems.
The callback listeners within the Google Mobile Ad SDK have been revised to improve your apps’ ability to react to reward events. Ad reward event handlers have been placed in their own distinct class, separate from full screen ad event listeners. Now you can keep your screen management logic separate from your game reward systems, and use a single full screen content event handler implementation for both rewarded and interstitial ad types.
Also, whereas the now deprecated AdMob SDK provided only one global listener for rewarded ads, you can now set a listener for each instance of rewarded ad that you load. This allows you to install varying listener behaviors depending on which scene your users earned their reward instead of having to track that state information yourself.