Building intelligent features into your apps can be complex. Testing models, building prompts, and debugging your solution are all real challenges when building apps powered by large language models.
We built Genkit to help you with these challenges. Genkit is an open-source framework with built-in tooling and observability. Together, these capabilities provide both a unified API to build your app using a variety of AI models from Google, OpenAI, Anthropic, and open models through Ollama, etc. While you’re building the app, you’ll also get the tooling needed to help you iterate quickly on your solution and debug common problems, as well as the observability you need to monitor your app in production once you deploy to Google Cloud or Firebase.
Earlier this year, we announced our stable release of Genkit for Node.js, and today we’re thrilled to announce the expansion of Genkit’s language support with Genkit for Python (Alpha) and Go (Beta).
These new SDKs bring Genkit’s powerful capabilities to even more developer ecosystems:
- Genkit for Python (Alpha) is ideal for experimentation and early development. The API may evolve rapidly as we incorporate your feedback, but all essential capabilities are available to start building today.
- Genkit for Go (Beta) has a rich feature set, and increased reliability as it approaches production readiness.
Whether you’re using Node.js, Python, or Go, you can now leverage Genkit’s consistent tools and concepts to build sophisticated AI-powered features with robust primitives, integrated tooling, and an extensible plugin system that simplifies prototyping, building, and deploying AI workflows.
We’re also excited about partners that build plug-ins for Genkit such as Pinecone, Astra DB, Neo4j, AuthO, and others. This growing ecosystem of plugins significantly expands Genkit’s capabilities, letting you to seamlessly integrate a wide array of specialized tools and services into their AI-powered applications.
Genkit for Python (Alpha)
If you’re building with Python, Genkit has intuitive and concise syntax for working with AI capabilities. The Python SDK offers a clean, asynchronous API with strong typing support through Pydantic integration.
Structured output
Python’s data class capabilities, enhanced by Pydantic for schema definition, shine in this Genkit example for structured output. Notice how the RpgCharacter
class clearly defines the expected format, ensuring the structured_character_flow
consistently returns AI-generated data in this predictable structure.
from pydantic import BaseModel, Field
from genkit.ai import Genkit
from genkit.plugins.google_genai import GoogleAI
ai = Genkit(
plugins=[GoogleAI()],
model='googleai/gemini-2.0-flash',
)
class RpgCharacter(BaseModel):
name: str = Field(description='name of the character')
backstory: str = Field(description='backstory')
abilities: list[str] = Field(description='list of abilities (3-4)')
@ai.flow()
async def structured_character_flow(name: str) -> RpgCharacter:
"""Generates a character profile conforming to the schema."""
result = await ai.generate(
prompt=f'Generate an RPG character named {name}',
output_schema=RpgCharacter,
)
return result.output
Agentive tool use
Genkit is also designed with intuitive and concise syntax for defining tools, as illustrated in this example. Leveraging decorators and Pydantic for data modeling, creating a tool like convert_currency
and integrating it into an asynchronous flow becomes straightforward.
# Imports and initialization omitted for brevity
class ConversionInput(BaseModel):
amount: float
@ai.tool()
async def convert_currency(input: ConversionInput) -> float:
"""Use this tool to convert USD to EUR"""
print(f"Converting {input.amount} USD...")
return input.amount * 0.92
@ai.flow()
async def currency_conversion_flow(amount: float) -> str:
"""Asks the model to convert currency, potentially using the tool."""
response = await ai.generate(
prompt=f'How much is {amount} USD in EUR?',
tools=[convert_currency],
)
return response.text()
Available Python Plugins
- Google GenAI: Access Google’s Gemini, Imagen, embedding models from Google AI Studio or Google Cloud Vertex AI.
- Ollama: Run open models like Gemma, Llama, and Mistral locally.
- Flask Integration: Easily serve your Genkit flows via a Flask web server.
- Firestore: Ground your generations with data retrieved using Firestore vector search.
Genkit for Go (Beta)
If you’re building with Go, Genkit offers a robust and explicit API with strong type safety, making it suitable for building scalable applications approaching production readiness.
Structured output
This Go snippet illustrates Genkit’s approach to guaranteeing structured output by explicitly defining a RpgCharacter
struct and using ai.WithOutputType
. Observe how the characterPrompt is configured with the desired output type, ensuring the structuredCharacterFlow
reliably produces data conforming to the specified schema.
package main
import (
"context"
"log"
"net/http"
"github.com/firebase/genkit/go/ai"
"github.com/firebase/genkit/go/genkit"
"github.com/firebase/genkit/go/plugins/googlegenai"
"github.com/firebase/genkit/go/server"
)
type RpgCharacter struct {
Name string `json:"name"`
Backstory string `json:"backstory"`
Abilities []string `json:"abilities"`
}
func main() {
ctx := context.Background()
g, err := genkit.Init(ctx,
genkit.WithPlugins(&googlegenai.GoogleAI{}),
genkit.WithDefaultModel("googleai/gemini-2.0-flash"),
)
if err != nil {
log.Fatalf("Genkit init failed: %v", err)
}
characterPrompt, _ := genkit.DefinePrompt(g, "characterPrompt",
ai.WithPrompt("Generate RPG character named {{name}}..."),
ai.WithInputType(RpgCharacter{Name: "Legolas"}) // Defaults to "Legolas".
ai.WithOutputType(RpgCharacter{}),
)
genkit.DefineFlow(g, "structuredCharacterFlow",
func(ctx context.Context, name string) (*RpgCharacter, error) {
resp, err := characterPrompt.Execute(ctx, ai.WithInput(RpgCharacter{Name: name}))
if err != nil {
return nil, err
}
var character RpgCharacter
if err := resp.Output(&character); err != nil {
return nil, err
}
return &character, nil
},
)
mux := http.NewServeMux()
for _, flow := range genkit.ListFlows(g) {
mux.HandleFunc("POST /"+flow.Name(), genkit.Handler(flow))
}
log.Fatal(server.Start(ctx, "127.0.0.1:8080", mux))
}
Run the Go app and call your flow using:
curl -X POST localhost:8080/structuredCharacterFlow \
-H "Content-Type: application/json" \
-d '{"data": "Elara"}'
Agentive tool use
Genkit offers a robust and explicit way to define and integrate custom tools into your AI workflows, as shown below. This example clearly demonstrates the registration of the convertCurrencyTool
and its use within the convertCurrencyFlow
, emphasizing Go’s type safety and suitability for building scalable applications.
// Imports omitted for brevity
func main() {
ctx := context.Background()
g, err := genkit.Init(ctx, genkit.WithPlugins(&googlegenai.GoogleAI{}))
if err != nil {
log.Fatalf("Genkit failed to initialize: %v", err)
}
convertCurrencyTool := genkit.DefineTool(g, "convertCurrencyTool",
"Use this tool to convert USD to EUR",
func(ctx *ai.ToolContext, amount float64) (float64, error) {
log.Printf("Converting %f USD", amount)
return amount * 0.92, nil
},
)
flow := genkit.DefineFlow(g, "convertCurrencyFlow",
func(ctx context.Context, amount float64) (string, error) {
return genkit.GenerateText(ctx, g,
ai.WithModelName("googleai/gemini-2.0-flash"),
ai.WithPrompt("How much is %f USD in EUR?", amount),
ai.WithTools(convertCurrencyTool),
)
},
)
mux := http.NewServeMux()
mux.HandleFunc("POST /convertCurrencyFlow", genkit.Handler(flow))
log.Fatal(server.Start(ctx, "127.0.0.1:8080", mux))
}
Run the Go app and call your flow:
curl -X POST localhost:8080/convertCurrencyFlow \
-H "Content-Type: application/json" \
-d '{"data": 190.00}'
Available Go Plugins
- Google GenAI: Access Gemini models and embeddings.
- Google Cloud Vertex AI: Integrate with Google Cloud’s AI platform.
- Ollama: Run open models locally.
- Pinecone: Connect to Pinecone’s vector database.
- pgvector: Integrate with PostgreSQL vector storage.
- Google Cloud Telemetry: Export observability data.
Developer tooling for rapid iteration
Building AI applications involves unique challenges. Genkit provides dedicated tooling—consistent across Python, Go, and Node.js—to accelerate testing and development.
Our CLI and intuitive browser-based Developer UI help you:
- Experiment Faster: Interactively run and iterate on your flows, prompts, and tools in dedicated playgrounds within the UI
- Debug Efficiently: Visualize detailed execution traces, inspect inputs/outputs (including structured data), view logs, and understand exactly how your flows are executing
- Evaluate Confidently: Test your AI workflows against predefined datasets and analyze scored metrics to ensure quality and reliability
Launch the Developer UI by running your app like so:
- Go:
genkit start -- go run main.go
- Python:
genkit start -- python main.py
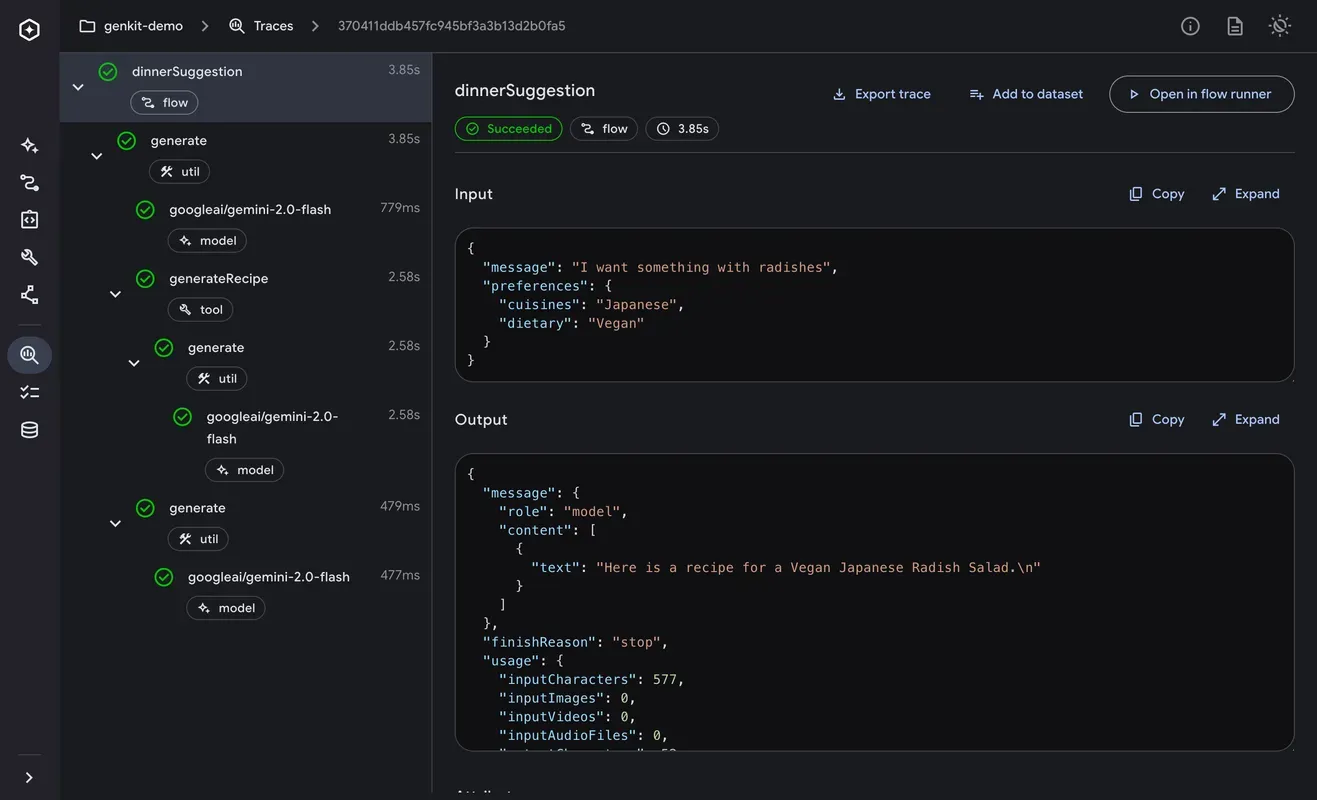
Get started
Ready to build your next AI-powered application? Explore our comprehensive documentation and resources:
- Python: Get Started with Genkit for Python (Alpha - Great for experimentation!)
- Go: Get Started with Genkit for Go (Beta - Maturing towards production-readiness!)
What’s next?
We are committed to continuously evolving and improving Genkit across all supported languages. We are actively working towards bringing Genkit for Python and Go to feature parity with Genkit for Node.js, as well as embracing language-specific benefits and integration.
Got questions or feedback? Join us on our Discord server, or Stack Overflow. For feature requests and bug reports, hit us up on the GitHub issue tracker.
Join our community, share your feedback, and help shape the future of Genkit! Whether you’re developing in Python or Go, now is the perfect time to explore the possibilities of building intelligent, agentive applications with Genkit.
Happy coding, and we look forward to seeing what you create!